Basic Setup
基本の準備
Swift is designed to provide seamless compatibility with Cocoa and Objective-C. You can use Objective-C APIs in Swift, and you can use Swift APIs in Objective-C. This makes Swift an easy, convenient, and powerful tool to integrate into your development workflow.
スウィフトは、ココアとObjective-Cに継ぎ目のない互換性を提供するように設計されます。あなたはObjective-CのAPIをスウィフトにおいて使うことができます、そしてあなたはスウィフト APIをObjective-Cにおいて使うことができます。これは、スウィフトを簡単で、便利で、強力なツールにして、あなたの開発ワークフローへと統合させます。
This guide covers three important aspects of Swift and Objective-C compatibility that you can use to your advantage when developing Cocoa apps:
このガイドは、この互換性の3つの重要な面を扱います、それは、あなたがココア・アプリを開発するときあなたの強みとして使うことができるものです:
Interoperability lets you interface between Swift and Objective-C code, allowing you to use Swift classes in Objective-C and to take advantage of familiar Cocoa classes, patterns, and practices when writing Swift code.
相互運用性は、あなたにスウィフトとObjective-Cコードの間を円滑に相互運用させて、スウィフトクラスをObjective-Cにおいて使用できるように、そしてスウィフトコードを書く場合には、よく知られているココア・クラス、パターン、および慣行を利用できるようにします。Mix and match allows you to create mixed-language apps containing both Swift and Objective-C files that can communicate with each other.
混合と適合は、あなたにお互いと通信することができるスウィフトとObjective-Cファイルを含んでいる混合言語アプリを作成することを可能にします。Migration from existing Objective-C code to Swift is made easy with interoperability and mix and match, making it possible to replace parts of your Objective-C apps with the latest Swift features.
移行。既存のObjective-Cコードからスウィフトへの移行は、相互運用性および混合と適合によって簡単にされます、そして、あなたのObjective-Cアプリの一部を最新のスウィフト特徴で取り替えることを可能にします。
Before you get started learning about these features, you need a basic understanding of how to set up a Swift environment in which you can access Cocoa system frameworks.
あなたがこれらの特徴について学ぶことを始める前に、あなたは、そこにおいてあなたがココア・システム・フレームワークにアクセスすることができるところの、スウィフト環境を設定する方法の基本的な理解が必要です。
Setting Up Your Swift Environment
あなたのスウィフト環境を設定する
To start experimenting with Cocoa app development using Swift, create a new Swift project from one of the provided Xcode templates.
スウィフトを使うCocoaアプリ開発の実験を始めるために、提供されるXcodeテンプレートの1つから新規スウィフトプロジェクトを作成してください。
To create a Swift project in Xcode
Xcodeにおいてスウィフトプロジェクトを作成します
Choose File > New > Project > (iOS, watchOS, tvOS, or macOS) > Application > your template of choice.
「ファイル」>「新規」>「プロジェクト」>(iOS、watchOS、tvOS、またはmacOS)>「アプリケーション」>あなたの選ぶひな形を選んでください。Click the Language pop-up menu and choose Swift.
「言語」ポップアップ・メニューをクリックして、スウィフトを選んでください。
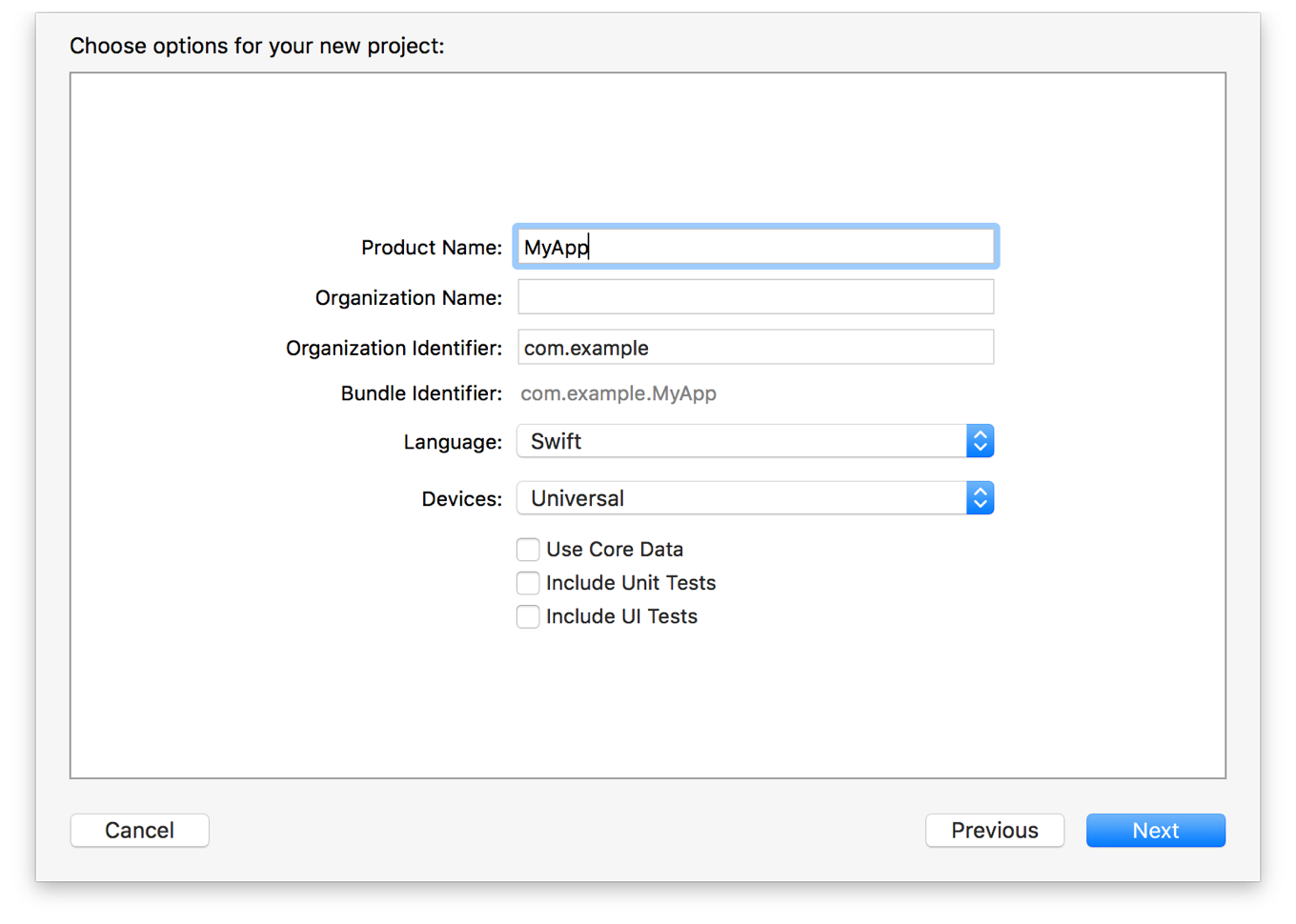
A Swift project’s structure is nearly identical to an Objective-C project, with one important distinction: Swift has no header files. There is no explicit delineation between the implementation and the interface—all of the information about a class, function, or constant resides in a single .swift
file. This is discussed in more detail in Swift and Objective-C in the Same Project.
スウィフトプロジェクトの構造は、Objective-Cプロジェクトにほとんど同一ですが、1つの重要な違いがあります:スウィフトには、ヘッダ・ファイルがありません。明確な境界線が実装とインタフェースの間にありません ― クラス、関数、または定数に関する全ての情報は1つの.swift
ファイルに属します。これは、より詳細に同じプロジェクト中のスウィフトとObjective-Cで議論されます。
From here, you can start experimenting by writing Swift code in the app delegate or a new Swift file you create by choosing File > New > File > (iOS, watchOS, tvOS, or macOS) > Source > Swift.
ここから、あなたはアプリデリケートまたは「ファイル」>「新規」>「ファイル」>(iOS、watchOS、tvOS、またはmacOS)>「ソース」>「Swift」を選んで作成する新規スウィフトファイルにおいてスウィフトコードを書くことで実験を始めることができます。
Understanding the Swift Import Process
スウィフトのインポートプロセスを理解する
After you have your Xcode project set up, you can import any framework from Cocoa or Cocoa Touch to start working with Objective-C from Swift.
あなたが、あなたのXcodeプロジェクトを設定した後は、あなたはどんなフレームワークでもCocoaまたはCocoa Touchからインポートすることで、スウィフトからObjective-Cを扱うことができます。
Any Objective-C framework or C library that supports modules can be imported directly into Swift. This includes all of the Objective-C system frameworks—such as Foundation, UIKit, and SpriteKit—as well as common C libraries supplied with the system. For example, to use Foundation APIs from a Swift file, add the following import statement to the top of the file:
モジュールをサポートするどんなObjective-CフレームワークまたはCライブラリでも、スウィフトに直接インポートされることができます。これは、Objective-Cシステムフレームワークの全て ― Foundation、UIKit、そしてSpriteKitなど ― それだけでなくシステムで供給される一般的なCライブラリをも含みます。例えば、Foundation APIをスウィフトファイルから利用するには、以下のインポート文をその ファイルの一番上に加えてください:
import Foundation
With this import statement, that Swift file can now access all of Foundation’s classes, protocols, methods, properties, and constants.
このインポート文で、このスウィフトファイルは今や全てのFoundationのクラス、プロトコル、メソッド、プロパティ、そして定数にアクセスできます。
The import process is straightforward. Objective-C frameworks vend APIs in header files. In Swift, those header files are compiled down to Objective-C modules, which are then imported into Swift as Swift APIs. The importing process determines how functions, classes, methods, and types declared in Objective-C code appear in Swift. For functions and methods, this process affects the types of their arguments and return values. For types, the process of importing can have the following effects:
インポートプロセスは単刀直入です。Objective-Cフレームワークは、ヘッダ・ファイルの中のAPIを販売します。スウィフトにおいて、それらのヘッダ・ファイルはObjective-Cモジュールに至るまでコンパイルされます、それらは、それからスウィフトにスウィフト APIとしてインポートされます。このインポートブロセスは、Objective-Cコードにおいて宣言した関数、クラス、メソッド、そして型が、スウィフトの中にどのように現れるかを決定します。関数とメソッドに対して、このプロセスは、それらの引数と戻り値の型に影響を及ぼします。型のために、インポートのプロセスは、以下の影響を与えることができます:
Remap certain Objective-C types to their equivalents in Swift, like
id
toAny
特定のObjective-C型をそれらのスウィフトでの等価物にリマップ(関数を別のキーに割り当てる)します、id
をAny
に、のようにRemap certain Objective-C core types to their alternatives in Swift, like
NSString
toString
特定のObjective-Cコア型をスウィフトでのそれらの代替物にリマップします、NSString
をString
へ、のようにRemap certain Objective-C concepts to matching concepts in Swift, like pointers to optionals
特定のObjective-C概念をスウィフトでの匹敵する概念にリマップします、ポインターをオプショナルへ、のように
For more information on using Objective-C in Swift, see Interacting with Objective-C APIs.
Objective-Cをスウィフトにおいて使うことに関するさらなる情報のためにObjective-C APIとの相互作用を見てください。
The model for importing Swift into Objective-C is similar to the one used for importing Objective-C into Swift. Swift vends its APIs—such as from a framework—as Swift modules. Alongside these Swift modules are generated Objective-C headers. These headers vend the APIs that can be mapped back to Objective-C. Some Swift APIs do not map back to Objective-C because they leverage language features that are not available in Objective-C.
スウィフトをObjective-Cにインポートすることの原型は、Objective-Cをスウィフトにインポートするために使われるものに似ています。スウィフトは、そのAPIを ― 例えばフレームワークから ― スウィフトモジュールとして売り渡します。それと並んでこれらのスウィフトモジュールは、Objective-Cヘッダを生成されます。これらのヘッダは、Objective-Cに逆にマップされることができるAPIを売り歩きます。スウィフト若干のAPIは、Objective-Cへと逆にマップされません、なぜならそれらがObjective-Cで利用できない言語機能に影響を及ぼすからです。
For more information on using Swift in Objective-C, see Swift and Objective-C in the Same Project.
Objective-Cでスウィフトを使うことの詳細については、同じプロジェクト中のスウィフトとObjective-Cを見てください。
Copyright © 2018 Apple Inc. All rights reserved. Terms of Use | Privacy Policy | Updated: 2018-03-29