Properties¶ プロパティ¶
Properties associate values with a particular class, structure, or enumeration. Stored properties store constant and variable values as part of an instance, whereas computed properties calculate (rather than store) a value. Computed properties are provided by classes, structures, and enumerations. Stored properties are provided only by classes and structures. プロパティは、いくつかの値を特定のクラス、構造体、または列挙と結びつけます。格納プロパティは、あるインスタンスの一部として定数と変数の値を格納します、一方で、計算プロパティは、値を(たくわえておくのではなく)計算します。計算プロパティは、クラス、構造体、および列挙で提供されます。格納プロパティは、クラスと構造体だけで提供されます。
Stored and computed properties are usually associated with instances of a particular type. However, properties can also be associated with the type itself. Such properties are known as type properties. 格納されるもしくは計算されるプロパティは、通常は特定の型のインスタンスに結び付けられます。しかし、プロパティはまた、型それ自体に結び付けられることができます。そのようなプロパティは、型プロパティとして知られています。
In addition, you can define property observers to monitor changes in a property’s value, which you can respond to with custom actions. Property observers can be added to stored properties you define yourself, and also to properties that a subclass inherits from its superclass. それに加えて、あなたはプロパティオブザーバーを定義して、あるプロパティの値の変化を監視することができて、あなたはあつらえの動作でそれに応答することができます。プロパティオブザーバーは、あなたがあなた自身で定義する格納プロパティに、そのうえにサブクラスがそのスーパークラスから継承するプロパティにも加えられることができます。
You can also use a property wrapper to reuse code in the getter and setter of multiple properties. あなたはまたプロパティラッパーを使用して、複数のプロパティのゲッターとセッターでコードを再利用できます。
Stored Properties¶ 格納プロパティ¶
In its simplest form, a stored property is a constant or variable that’s stored as part of an instance of a particular class or structure. Stored properties can be either variable stored properties (introduced by the var
keyword) or constant stored properties (introduced by the let
keyword).
その最も単純な形式で、格納プロパティは、特定のクラスまたは構造体のあるひとつのインスタンスの一部として格納される定数または変数です。格納プロパティは、変数格納プロパティ(var
キーワードによって導入される)か定数格納プロパティ(let
キーワードによって導入される)であることができます。
You can provide a default value for a stored property as part of its definition, as described in Default Property Values. You can also set and modify the initial value for a stored property during initialization. This is true even for constant stored properties, as described in Assigning Constant Properties During Initialization. 省略時のプロパティ値で記述されるように、あなたは格納プロパティのために省略時の値をその定義の一部として用意することができます。あなたは、また、初期化のときに格納プロパティに対して最初の値を設定および修正することができます。初期化の間に定数プロパティを割り当てるで記述されるように、これは定数格納プロパティにさえあてはまります。
The example below defines a structure called FixedLengthRange
, which describes a range of integers whose range length can’t be changed after it’s created:
以下の例はFixedLengthRange
と呼ばれる構造体を定義します、それは、それが作成された後に範囲長が変更されることが出来ない、整数からなるある範囲を記述します:
- struct FixedLengthRange {
- var firstValue: Int
- let length: Int
- }
- var rangeOfThreeItems = FixedLengthRange(firstValue: 0, length: 3)
- // the range represents integer values 0, 1, and 2(範囲は、整数値0、1、そして2を表します)
- rangeOfThreeItems.firstValue = 6
- // the range now represents integer values 6, 7, and 8(範囲は、現在は整数値6、7、そして8を表します)
Instances of FixedLengthRange
have a variable stored property called firstValue
and a constant stored property called length
. In the example above, length
is initialized when the new range is created and can’t be changed thereafter, because it’s a constant property.
FixedLengthRange
のインスタンスはfirstValue
と呼ばれる変数格納プロパティとlength
と呼ばれる定数格納プロパティをもちます。上の例で、length
は新しい範囲が作成される時に初期化されます、そしてその後は変更されることが出来ません、なぜならそれが変数プロパティだからです。
Stored Properties of Constant Structure Instances¶ 定数構造体インスタンスの格納プロパティ¶
If you create an instance of a structure and assign that instance to a constant, you can’t modify the instance’s properties, even if they were declared as variable properties: あなたが構造体のインスタンスをつくって、そのインスタンスを定数に代入するならば、あなたはそのインスタンスのもつプロパティを、たとえそれらが変数プロパティとして宣言されたとしても、修正することはできません:
- let rangeOfFourItems = FixedLengthRange(firstValue: 0, length: 4)
- // this range represents integer values 0, 1, 2, and 3(この範囲構造体、整数値0、1、2、そして3を表します)
- rangeOfFourItems.firstValue = 6
- // this will report an error, even though firstValue is a variable property(これはエラーを報告します、たとえfirstValueが変数プロパティであるとしてもです)
Because rangeOfFourItems
is declared as a constant (with the let
keyword), it isn’t possible to change its firstValue
property, even though firstValue
is a variable property.
rangeOfFourItems
が定数(let
キーワードを使って)として宣言されるので、たとえfirstValue
が変数プロパティであるとしても、そのfirstValue
プロパティを変えることは可能ではありません。
This behavior is due to structures being value types. When an instance of a value type is marked as a constant, so are all of its properties. この挙動は、この構造体が値型であるためです。値型のインスタンスが定数として印される時は、そのプロパティの全てがそうです。
The same isn’t true for classes, which are reference types. If you assign an instance of a reference type to a constant, you can still change that instance’s variable properties. 同じことはクラスにはあてはまりません、それは参照型です。あなたが参照型のインスタンスを定数に代入するならば、あなたは依然としてそのインスタンスの変数プロパティを変えることができます。
Lazy Stored Properties¶ 遅延格納プロパティ¶
A lazy stored property is a property whose initial value isn’t calculated until the first time it’s used. You indicate a lazy stored property by writing the lazy
modifier before its declaration.
遅延格納プロパティは、初めてそれが使われるまで、初期値が計算されないプロパティです。あなたは、lazy
修飾子をその宣言の前に書くことによって遅延格納プロパティであることを示します。
Note 注意
You must always declare a lazy property as a variable (with the var
keyword), because its initial value might not be retrieved until after instance initialization completes. Constant properties must always have a value before initialization completes, and therefore can’t be declared as lazy.
あなたは常に遅延プロパティを変数として(var
キーワードを使って)宣言しなければなりません、なぜならその最初の値はインスタンスの初期化が完了する後まで取り出されないかもしれないからです。定数プロパティは常に値を、初期化が完了する前に持たなければなりません、それゆえに遅延であると宣言されることが出来ません。
Lazy properties are useful when the initial value for a property is dependent on outside factors whose values aren’t known until after an instance’s initialization is complete. Lazy properties are also useful when the initial value for a property requires complex or computationally expensive setup that shouldn’t be performed unless or until it’s needed. 遅延プロパティが役に立つのは、プロパティのための最初の値がインスタンスの初期化が終了する後まで値が知られない外部の要因に依存しているときです。遅延プロパティはまた、プロパティのための最初の値が、それが必要でないなら、あるいは必要になるまで、実行されるべきではない複雑なまたはコンピュータ処理的に高くつく準備を必要とするときに役に立ちます。
The example below uses a lazy stored property to avoid unnecessary initialization of a complex class. This example defines two classes called DataImporter
and DataManager
, neither of which is shown in full:
下の例は、ある複雑なクラスの必要でない初期化を避けるために遅延格納プロパティを使います。この例は、DataImporter
とDataManager
と呼ばれる2つのクラスを定義します、そのどちらも全部は示されません:
- class DataImporter {
- /*
- DataImporter is a class to import data from an external file.(DataImporterは、データを外部ファイルからインポートするクラスです。)
- The class is assumed to take a nontrivial amount of time to initialize.(このクラスは、瑣末でない量の時間が初期化にかかると仮定されます。)
- */
- var filename = "data.txt"
- // the DataImporter class would provide data importing functionality here(DataImporterクラスは、データをインポートする機能性をここで提供します)
- }
- class DataManager {
- lazy var importer = DataImporter()
- var data: [String] = []
- // the DataManager class would provide data management functionality here(DataManagerクラスは、データ管理の機能性をここで提供します)
- }
- let manager = DataManager()
- manager.data.append("Some data")
- manager.data.append("Some more data")
- // the DataImporter instance for the importer property hasn't yet been created(importerプロパティのためのDataImporterインスタンスは、まだ作成されていません)
The DataManager
class has a stored property called data
, which is initialized with a new, empty array of String
values. Although the rest of its functionality isn’t shown, the purpose of this DataManager
class is to manage and provide access to this array of String
data.
DataManager
クラスはdata
と呼ばれる格納プロパティを持ちます、それは、String
値の、新規の、空の配列で初期化されます。その機能性の残りの部分は示されないけれども、このDataManager
クラスの目的はこのString
データの配列を管理して、アクセス提供することになっています。
Part of the functionality of the DataManager
class is the ability to import data from a file. This functionality is provided by the DataImporter
class, which is assumed to take a nontrivial amount of time to initialize. This might be because a DataImporter
instance needs to open a file and read its contents into memory when the DataImporter
instance is initialized.
DataManager
クラスの機能性の一部は、データをファイルからインポートする能力です。この機能性はDataImporter
クラスによって提供されます、それは、初期化するために瑣末でない量の時間がかかると仮定されます。これは、DataImporter
インスタンスが初期化されるとき、DataImporter
インスタンスがファイルを開いてメモリーにその内容を読む必要があるからでしょう。
Because it’s possible for a DataManager
instance to manage its data without ever importing data from a file, DataManager
doesn’t create a new DataImporter
instance when the DataManager
itself is created. Instead, it makes more sense to create the DataImporter
instance if and when it’s first used.
DataManager
インスタンスにはそれのデータを管理することがファイルからデータを一度もインポートせずに可能なので、DataManager
は新しいDataImporter
インスタンスの作成をDataManager
それ自身が作成される時に行いません。その代わりに、それが最初に必要とされる時になればDataImporter
を作成するほうが筋が通ります。
Because it’s marked with the lazy
modifier, the DataImporter
instance for the importer
property is only created when the importer
property is first accessed, such as when its filename
property is queried:
それがlazy
修飾子という特徴をもつので、importer
プロパティのためのDataImporter
インスタンスは、importer
プロパティが最初にアクセスされるsgにだけ作成されます、例えばそのfilename
プロパティがたずねられるときなど:
- print(manager.importer.filename)
- // the DataImporter instance for the importer property has now been created(importerプロパティのためのDataImporterインスタンスが、今つくられました)
- // Prints "data.txt"(「data.txt」を出力します)
Note 注意
If a property marked with the lazy
modifier is accessed by multiple threads simultaneously and the property hasn’t yet been initialized, there’s no guarantee that the property will be initialized only once.
lazy
修飾子で印されるプロパティが複数のスレッドによって同時にアクセスされ、そのプロパティがその時まだ初期化されないならば、そのプロパティがただ一度だけ初期化されるとは保障されません。
Stored Properties and Instance Variables¶ 格納プロパティとインスタンス変数¶
If you have experience with Objective-C, you may know that it provides two ways to store values and references as part of a class instance. In addition to properties, you can use instance variables as a backing store for the values stored in a property. あなたがObjective-Cでの経験を持つならば、あなたはそれがクラスインスタンスの一部として値や参照を格納するために2つの方法を提供するということを知っているかもしれません。プロパティに加えて、あなたはインスタンス変数をプロパティに格納される値に対する支援外部記憶として使うことができます。
Swift unifies these concepts into a single property declaration. A Swift property doesn’t have a corresponding instance variable, and the backing store for a property isn’t accessed directly. This approach avoids confusion about how the value is accessed in different contexts and simplifies the property’s declaration into a single, definitive statement. All information about the property—including its name, type, and memory management characteristics—is defined in a single location as part of the type’s definition. スウィフトは、これらの概念をだだ1つのプロパティ宣言に統一します。スウィフトのプロパティには対応するインスタンス変数がありません、そしてプロパティのための支援外部記憶は直接にアクセスされません。この取り組み方は、値が異なる文脈においてアクセスされる方法についての混乱を避け、プロパティの宣言をただ1つの、決定的な文に単純化します。プロパティに関する全ての情報は ― その名前、型、そしてメモリ管理などの特徴を含めて ― その型の定義の一部として1つの場所で定義されます。
Computed Properties¶ 計算プロパティ¶
In addition to stored properties, classes, structures, and enumerations can define computed properties, which don’t actually store a value. Instead, they provide a getter and an optional setter to retrieve and set other properties and values indirectly. 格納プロパティに加えて、クラス、構造体、および列挙は計算プロパティを定義することができます、それは、実際に値を格納しません。その代わりに、それらは他のプロパティや値を間接的に取得したり設定したりするためにゲッターと任意のセッターを提供します。
- struct Point {
- var x = 0.0, y = 0.0
- }
- struct Size {
- var width = 0.0, height = 0.0
- }
- struct Rect {
- var origin = Point()
- var size = Size()
- var center: Point {
- get {
- let centerX = origin.x + (size.width / 2)
- let centerY = origin.y + (size.height / 2)
- return Point(x: centerX, y: centerY)
- }
- set(newCenter) {
- origin.x = newCenter.x - (size.width / 2)
- origin.y = newCenter.y - (size.height / 2)
- }
- }
- }
- var square = Rect(origin: Point(x: 0.0, y: 0.0),
- size: Size(width: 10.0, height: 10.0))
- let initialSquareCenter = square.center
- // initialSquareCenter is at (5.0, 5.0)
- square.center = Point(x: 15.0, y: 15.0)
- print("square.origin is now at (\(square.origin.x), \(square.origin.y))")
- // Prints "square.origin is now at (10.0, 10.0)"(「square.originは、現在(10.0, 10.0)です」を出力します))
This example defines three structures for working with geometric shapes: この例は、幾何学形を扱うために3つの構造体を定義します:
Point
encapsulates the x- and y-coordinate of a point.Point
は、ある地点のxおよひy座標をカプセル化します。Size
encapsulates awidth
and aheight
.Size
は、width
とheight
をカプセル化します。Rect
defines a rectangle by an origin point and a size.Rect
は、原点と大きさによって長方形を定義します。
The Rect
structure also provides a computed property called center
. The current center position of a Rect
can always be determined from its origin
and size
, and so you don’t need to store the center point as an explicit Point
value. Instead, Rect
defines a custom getter and setter for a computed variable called center
, to enable you to work with the rectangle’s center
as if it were a real stored property.
Rect
構造体はまた、center
(中心)と呼ばれる計算プロパティを提供します。Rect
の現在の中心位置は常にそのorigin
とsize
から決定されることができます、なのであなたは中心点をわざわざPoint
値として格納する必要ばありません。その代わりに、Rect
は、center
と呼ばれる計算される変数のためにあつらえのゲッターとセッターを定義して、矩形のcenter
をそれがまったく格納プロパティであるかのようにあなたが扱うことができるようにします。
The example above creates a new Rect
variable called square
. The square
variable is initialized with an origin point of (0, 0)
, and a width and height of 10
. This square is represented by the light green square in the diagram below.
上の例は、square
と呼ばれる新しいRect
変数をつくります。square
変数は、(0, 0)
の原点、そして10
の幅と高さで初期化されます。この正方形は、下の図において明るい緑の正方形で表されます。
The square
variable’s center
property is then accessed through dot syntax (square.center
), which causes the getter for center
to be called, to retrieve the current property value. Rather than returning an existing value, the getter actually calculates and returns a new Point
to represent the center of the square. As can be seen above, the getter correctly returns a center point of (5, 5)
.
square
変数のcenter
プロパティは、それからドット構文(square.center
)を通してアクセスされます、それによってcenter
のためのゲッターが呼び出されて、現在のプロパティ値を取り出すことになります。既存の値を返すのではなく、このゲッターは正方形の中心を表すために実際に計算して新しいPoint
を返します。上で見られるように、ゲッターは正しく中心点(5, 5)
を返します。
The center
property is then set to a new value of (15, 15)
, which moves the square up and to the right, to the new position shown by the dark green square in the diagram below. Setting the center
property calls the setter for center
, which modifies the x
and y
values of the stored origin
property, and moves the square to its new position.
center
プロパティは、それから新しい値(15, 15)
に設定されます、それは、正方形を上にそして右に、下記の図において暗い緑の正方形によって示される新しい位置へと動かします。center
プロパティを設定することは、center
のためのセッターを呼び出します、そしてそれは、格納されたorigin
プロパティのx
とy
値を修正して、正方形をその新しい位置へと動かします。
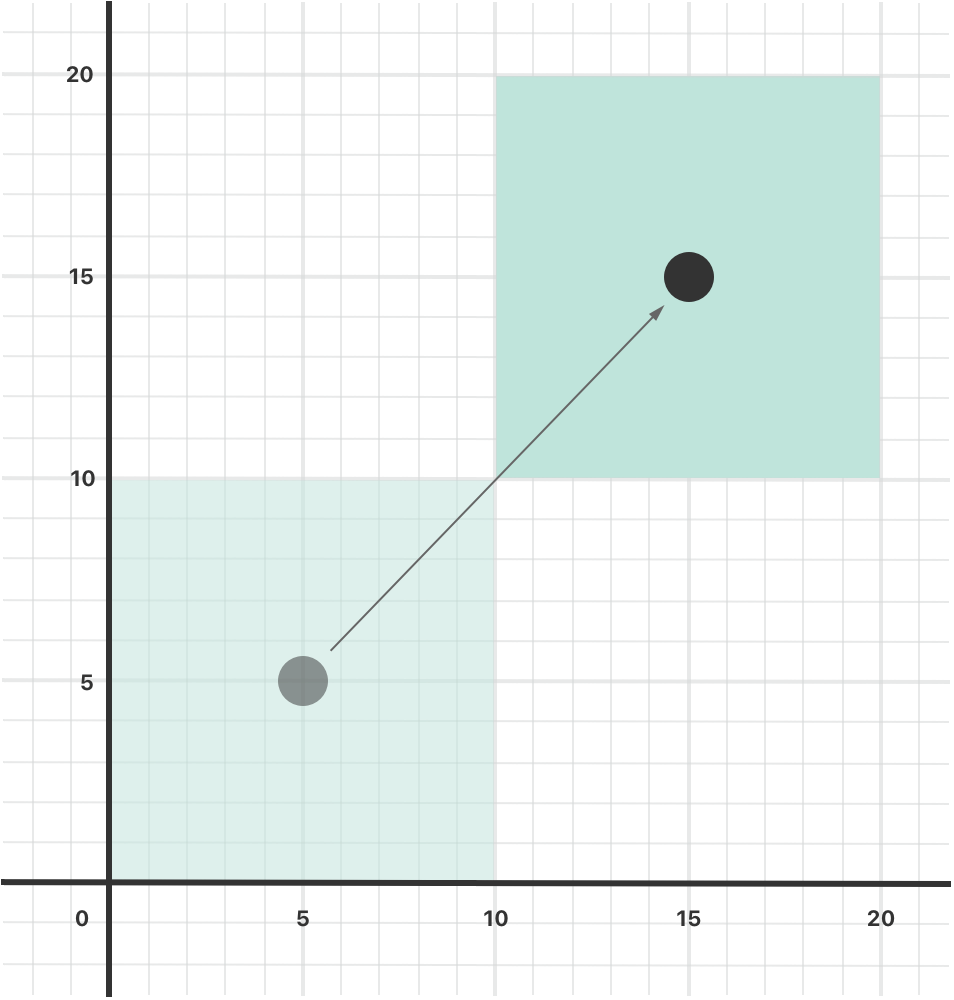
Shorthand Setter Declaration¶ 短縮形セッター宣言¶
If a computed property’s setter doesn’t define a name for the new value to be set, a default name of newValue
is used. Here’s an alternative version of the Rect
structure that takes advantage of this shorthand notation:
計算プロパティのもつセッターがその設定される新しい値に名前を定義しないならば、newValue
の省略時の値が使われます。ここにRect
構造体の代替のバージョンがあります、それはこの短縮形表記法を利用します:
- struct AlternativeRect {
- var origin = Point()
- var size = Size()
- var center: Point {
- get {
- let centerX = origin.x + (size.width / 2)
- let centerY = origin.y + (size.height / 2)
- return Point(x: centerX, y: centerY)
- }
- set {
- origin.x = newValue.x - (size.width / 2)
- origin.y = newValue.y - (size.height / 2)
- }
- }
- }
Shorthand Getter Declaration¶ 略記ゲッター宣言¶
If the entire body of a getter is a single expression, the getter implicitly returns that expression. Here’s an another version of the Rect
structure that takes advantage of this shorthand notation and the shorthand notation for setters:
ゲッターの全本文が単一の式ならば、ゲッターは暗黙的にその式を返します。ここにRect
構造体の別のバージョンがあります、それはこの省略形表記法とセッターに対する省略形表記法を利用します:
- struct CompactRect {
- var origin = Point()
- var size = Size()
- var center: Point {
- get {
- Point(x: origin.x + (size.width / 2),
- y: origin.y + (size.height / 2))
- }
- set {
- origin.x = newValue.x - (size.width / 2)
- origin.y = newValue.y - (size.height / 2)
- }
- }
- }
Omitting the return
from a getter follows the same rules as omitting return
from a function, as described in Functions With an Implicit Return.
return
をゲッターから省くことは、return
を関数から省くのと同じ規則に従います、暗黙的なreturnをもつ関数で記述されるように。
Read-Only Computed Properties¶ 読み出し専用の計算プロパティ¶
A computed property with a getter but no setter is known as a read-only computed property. A read-only computed property always returns a value, and can be accessed through dot syntax, but can’t be set to a different value. ゲッターを持つ、しかしセッターはない計算プロパティは、読み出し専用の計算プロパティとして知られています。読み出し専用の計算プロパティは常にある値を返します、そしてドット構文を通してアクセスされることができますが、異なる値に設定されることができません。
Note 注意
You must declare computed properties—including read-only computed properties—as variable properties with the var
keyword, because their value isn’t fixed. The let
keyword is only used for constant properties, to indicate that their values can’t be changed once they’re set as part of instance initialization.
あなたは、計算プロパティ ― 読み出し専用の計算プロパティを含む ― をvar
キーワードをつかって変数プロパティとして宣言しなければなりません、なぜならそれらの値は固定されないからです。let
キーワードは定数プロパティのためにだけ使われます、そして一旦それがインスタンス初期化の一部として設定されるならば、その値が変わることができないことを示します。
You can simplify the declaration of a read-only computed property by removing the get
keyword and its braces:
あなたは、読み出し専用の計算プロパティの宣言をget
キーワードとそれの波括弧を取り除くことによって単純化することができます:
- struct Cuboid {
- var width = 0.0, height = 0.0, depth = 0.0
- var volume: Double {
- return width * height * depth
- }
- }
- let fourByFiveByTwo = Cuboid(width: 4.0, height: 5.0, depth: 2.0)
- print("the volume of fourByFiveByTwo is \(fourByFiveByTwo.volume)")
- // Prints "the volume of fourByFiveByTwo is 40.0"(「fourByFiveByTwoのボリュームは、40.0です」を出力します)
This example defines a new structure called Cuboid
, which represents a 3D rectangular box with width
, height
, and depth
properties. This structure also has a read-only computed property called volume
, which calculates and returns the current volume of the cuboid. It doesn’t make sense for volume
to be settable, because it would be ambiguous as to which values of width
, height
, and depth
should be used for a particular volume
value. Nonetheless, it’s useful for a Cuboid
to provide a read-only computed property to enable external users to discover its current calculated volume.
この例はCuboid
と呼ばれる新しい構造体を定義します、それは、width
、height
、そしてdepth
プロパティで3D矩形の箱を表します。この構造体はまた、volume
と呼ばれる読み出し専用の計算プロパティを持ちます、それは、現在の立方体の体積を計算して、返します。volume
が設定可能であることは意味をなしません、なぜなら特定のvolume
値に対してwidth
、height
、そしてdepth
にどの値が使われなければならないかはあいまいだからです。それでもなお、Cuboid
が外部のユーザーにその現在の計算された体積を見つけられるように読み出し専用の計算プロパティを提供することは、役に立ちます。
Property Observers¶ プロパティオブザーバー¶
Property observers observe and respond to changes in a property’s value. Property observers are called every time a property’s value is set, even if the new value is the same as the property’s current value. プロパティオブザーバーは、あるプロパティの値の変化を観察して、応答します。プロパティオブザーバーは、あるプロパティの値が設定されるたびに、たとえ新しい値がそのプロパティの現在の値と同じものであるとしても、呼ばれます。
You can add property observers in the following places: あなたは、プロパティオブザーバを以下の場所に追加できます:
- Stored properties that you define あなたが定義する格納プロパティ
- Stored properties that you inherit あなたが継承する格納プロパティ
- Computed properties that you inherit あなたが継承する計算プロパティ
For an inherited property, you add a property observer by overriding that property in a subclass. For a computed property that you define, use the property’s setter to observe and respond to value changes, instead of trying to create an observer. Overriding properties is described in Overriding. 継承されたプロパティに対して、あなたはプロパティオブザーバを、そのプロパティをサブクラスの中でオーバーライドすることによって追加します。あなたが定義する計算プロパティに対して、プロパティのもつセッターを使うことで値の変化を監視および応答してください、オブザーバを作成しようとするのではなく。プロパティのオーバーライドは、オーバーライドにおいて記述されます。
You have the option to define either or both of these observers on a property: あなたは、あるプロパティに関してこれらのオブザーバーのどちらかまたは両方とも定義する選択肢を持ちます:
willSet
is called just before the value is stored.willSet
は、値が格納される直前に呼ばれます。didSet
is called immediately after the new value is stored.didSet
は、新しい値が格納された直後に呼ばれます。
If you implement a willSet
observer, it’s passed the new property value as a constant parameter. You can specify a name for this parameter as part of your willSet
implementation. If you don’t write the parameter name and parentheses within your implementation, the parameter is made available with a default parameter name of newValue
.
あなたがwillSet
オブザーバーを実装するならば、それは新しいプロパティ値を定数パラメータとして渡されます。あなたは、あなたのwillSet
実装の一部としてこのパラメータに名前を指定することができます。あなたがパラメータ名と丸括弧をあなたの実装内で書かないならば、パラメータは省略時のパラメータ名のnewValue
を使って利用可能にされます。
Similarly, if you implement a didSet
observer, it’s passed a constant parameter containing the old property value. You can name the parameter or use the default parameter name of oldValue
. If you assign a value to a property within its own didSet
observer, the new value that you assign replaces the one that was just set.
同じように、あなたがdidSet
オブザーバーを実装するならば、それは古いプロパティ値を含んでいる定数パラメータを渡されます。あなたはパラメータに名をつけること、または省略時のパラメータ名のoldValue
を使うことが出来ます。あなたが独自のdidSet
オブザーバーの内部で値をプロパティに代入するならば、あなたが代入する新しい値はついさっき設定されたものを置き換えます。
Note 注意
The willSet
and didSet
observers of superclass properties are called when a property is set in a subclass initializer, after the superclass initializer has been called. They aren’t called while a class is setting its own properties, before the superclass initializer has been called.
スーパークラスプロパティのwillSet
とdidSet
オブザーバーは、スーパークラスイニシャライザが呼び出され終わった後、あるプロパティがサプクラスのイニシャライザにおいて設定される時に呼び出されます。それらは、スーパークラスイニシャライザが呼び出され終わる前、あるクラスがそれ自身のプロパティを設定している間には呼び出されません。
For more information about initializer delegation, see Initializer Delegation for Value Types and Initializer Delegation for Class Types. イニシャライザ委任についての更なる情報として、値型のためのイニシャライザ委任とクラス型のためのイニシャライザ委任を見てください。
Here’s an example of willSet
and didSet
in action. The example below defines a new class called StepCounter
, which tracks the total number of steps that a person takes while walking. This class might be used with input data from a pedometer or other step counter to keep track of a person’s exercise during their daily routine.
willSet
とdidSet
の作動する例が、ここにあります。下の例は、StepCounter
と呼ばれる新しいクラスを定義します、それは、ある人が歩いている間にとる総歩数を追跡します。このクラスは、万歩計または他の歩数計からの入力データとともに、ある個人の日課の運動の経過を追うために使われるかもしれません。
- class StepCounter {
- var totalSteps: Int = 0 {
- willSet(newTotalSteps) {
- print("About to set totalSteps to \(newTotalSteps)")
- }
- didSet {
- if totalSteps > oldValue {
- print("Added \(totalSteps - oldValue) steps")
- }
- }
- }
- }
- let stepCounter = StepCounter()
- stepCounter.totalSteps = 200
- // About to set totalSteps to 200(totalStepsを200に設定している)
- // Added 200 steps(200の歩数が加えられた)
- stepCounter.totalSteps = 360
- // About to set totalSteps to 360(totalStepsを360に設定している)
- // Added 160 steps(160の歩数が加えられた)
- stepCounter.totalSteps = 896
- // About to set totalSteps to 896(totalStepsを896に設定している)
- // Added 536 steps(536の歩数が加えられた)
The StepCounter
class declares a totalSteps
property of type Int
. This is a stored property with willSet
and didSet
observers.
StepCounter
クラスは、型Int
のtotalSteps
プロパティを宣言します。これは、willSet
とdidSet
オブザーバーを持つ格納プロパティです。
The willSet
and didSet
observers for totalSteps
are called whenever the property is assigned a new value. This is true even if the new value is the same as the current value.
totalSteps
のためのwillSet
とdidSet
オブザーバーは、プロパティが新しい値を代入されるときはいつでも呼ばれます。これは、たとえ新しい値が現在の値と同じものであるとしても当てはまります。
This example’s willSet
observer uses a custom parameter name of newTotalSteps
for the upcoming new value. In this example, it simply prints out the value that’s about to be set.
この例のwillSet
オブザーバーは、来るべき新しい値のためにあつらえのパラメータ名newTotalSteps
を使用します。この例では、それは単に設定されようとしている値を出力します。
The didSet
observer is called after the value of totalSteps
is updated. It compares the new value of totalSteps
against the old value. If the total number of steps has increased, a message is printed to indicate how many new steps have been taken. The didSet
observer doesn’t provide a custom parameter name for the old value, and the default name of oldValue
is used instead.
didSet
オブザーバーは、totalSteps
の値が更新された後に呼ばれます。それは、totalSteps
の新しい値をその古い値と比較します。総歩数が増加したならば、どれくらいの新たな歩数がとられたかについて示すためにメッセージが出力されます。didSet
オブザーバーはあつらえのパラメータ名を古い値のために用意しません、そして省略時の名前のoldValue
がその代わりに使われます。
Note 注意
If you pass a property that has observers to a function as an in-out parameter, the willSet
and didSet
observers are always called. This is because of the copy-in copy-out memory model for in-out parameters: The value is always written back to the property at the end of the function. For a detailed discussion of the behavior of in-out parameters, see In-Out Parameters.
あなたがオブザーバーを持つプロパティをある関数へin-outパラメータとして渡すならば、willSet
とdidSet
オブザーバーは常に呼び出されます。これは、in-outパラメータのための「コピーイン、コピーアウト」メモリーモデルが理由です:値は常に、その関数の終わりでプロパティへと書き込まれて戻されます。in-outパラメータの振る舞いの詳細な議論のために、in-outパラメータを見てください。
Property Wrappers¶ プロパティラッパー¶
A property wrapper adds a layer of separation between code that manages how a property is stored and the code that defines a property. For example, if you have properties that provide thread-safety checks or store their underlying data in a database, you have to write that code on every property. When you use a property wrapper, you write the management code once when you define the wrapper, and then reuse that management code by applying it to multiple properties. プロパティラッパーは、プロパティが格納される方法を管理するコードとプロパティを定義するコードとの間に分離のレイヤー(層)を加えます。例えば、あなたがスレッド安全検査を提供するまたはそれらの基礎をなすデータをデータベースに格納するプロパティそれらを持つならば、あなたはそのコードをあらゆるプロパティ上で書かなければなりません。あなたがプロパティラッパーを使う場合、あなたは管理コードを一度だけあなたがラッパーを定義する時に書きます、そしてそれからその管理コードを、それを複数のプロパティに適用することによって再利用します。
To define a property wrapper, you make a structure, enumeration, or class that defines a wrappedValue
property. In the code below, the TwelveOrLess
structure ensures that the value it wraps always contains a number less than or equal to 12. If you ask it to store a larger number, it stores 12 instead.
プロパティラッパーを定義するには、あなたはあるwrappedValue
プロパティを定義する構造体、列挙、またはクラスを作ります。下のコードにおいて、TwelveOrLess
構造体は、それがラップする値が常に12より少ないか等しいある数を含むことを保証します。あなたがそれにより大きな数を格納するように頼むならば、それは代わりに12を格納します。
- @propertyWrapper
- struct TwelveOrLess {
- private var number = 0
- var wrappedValue: Int {
- get { return number }
- set { number = min(newValue, 12) }
- }
- }
The setter ensures that new values are less than or equal to 12, and the getter returns the stored value. セッターは、新しい値が12より少ないかまたは等しいことを保証します、そしてゲッターは格納された値を返します。
Note 注意
The declaration for number
in the example above marks the variable as private
, which ensures number
is used only in the implementation of TwelveOrLess
. Code that’s written anywhere else accesses the value using the getter and setter for wrappedValue
, and can’t use number
directly. For information about private
, see Access Control.
number
に対する宣言は上の例において、変数をprivate
として印します、それはnumber
がTwelveOrLess
の実装においてのみ使われることを保証します。どこか他で書かれるコードは、wrappedValue
に対するゲッターとセッターを使って値にアクセスします、そしてnumber
を直接に使用できません。private
に対する情報として、アクセス制御を見てください。
You apply a wrapper to a property by writing the wrapper’s name before the property as an attribute. Here’s a structure that stores a rectangle that uses the TwelveOrLess
property wrapper to ensure its dimensions are always 12 or less:
あなたは、ラッパーのもつ名前をそのプロパティの前に属性として書くことによって、ラッパーをプロパティに適用します。ここにある構造体があります、それはある矩形を格納します、それはTwelveOrLess
プロパティラッパーを使うことでそれの寸法を常に12かそれ以下にします:
- struct SmallRectangle {
- @TwelveOrLess var height: Int
- @TwelveOrLess var width: Int
- }
- var rectangle = SmallRectangle()
- print(rectangle.height)
- // Prints "0"(「0」を出力します)
- rectangle.height = 10
- print(rectangle.height)
- // Prints "10"(「10」を出力します)
- rectangle.height = 24
- print(rectangle.height)
- // Prints "12"(「12」を出力します)
The height
and width
properties get their initial values from the definition of TwelveOrLess
, which sets TwelveOrLess.number
to zero. The setter in TwelveOrLess
treats 10 as a valid value so storing the number 10 in rectangle.height
proceeds as written. However, 24 is larger than TwelveOrLess
allows, so trying to store 24 end up setting rectangle.height
to 12 instead, the largest allowed value.
height
とwidth
プロパティは、それらの初期値をTwelveOrLess
の定義から取得します、それはTwelveOrLess.number
をゼロに設定します。TwelveOrLess
の中のセッターは、10を有効な値として扱うので、数10をrectangle.height
の中に格納することは、書き出されたとおりに起こります。しかしながら、24はTwelveOrLess
が許可するより大きいので、24を格納する試みは、rectangle.height
を代わりに12、最も大きな許可される値へと設定することに結局なります。
When you apply a wrapper to a property, the compiler synthesizes code that provides storage for the wrapper and code that provides access to the property through the wrapper. (The property wrapper is responsible for storing the wrapped value, so there’s no synthesized code for that.) You could write code that uses the behavior of a property wrapper, without taking advantage of the special attribute syntax. For example, here’s a version of SmallRectangle
from the previous code listing that wraps its properties in the TwelveOrLess
structure explicitly, instead of writing @TwelveOrLess
as an attribute:
あなたがあるラッパーをプロパティに適用する場合、コンパイラは、そのラッパーに対するストレージを提供するコードそしてそのラッパーに通してプロパティへのアクセスを提供するコードを合成します。(プロパティラッパーは、ラップ値を格納することに責任があります、それでそれに対する合成コードはありません。)あなたはプロパティラッパーの挙動を使うコードを、この特別な属性構文を利用することなく記述できます。例えば、ここに前のコード出力からのSmallRectangle
の変形版があります、それはそれのプロパティをTwelveOrLess
構造体の中に明示的にラップします、@TwelveOrLess
を属性として書く代わりに。
- struct SmallRectangle {
- private var _height = TwelveOrLess()
- private var _width = TwelveOrLess()
- var height: Int {
- get { return _height.wrappedValue }
- set { _height.wrappedValue = newValue }
- }
- var width: Int {
- get { return _width.wrappedValue }
- set { _width.wrappedValue = newValue }
- }
- }
The _height
and _width
properties store an instance of the property wrapper, TwelveOrLess
. The getter and setter for height
and width
wrap access to the wrappedValue
property.
_height
と_width
プロパティは、プロパティラッパー、TwelveOrLess
のインスタンスを格納します。height
とwidth
に対するゲッターとセッターは、wrappedValue
プロパティへのアクセスをラップします。
Setting Initial Values for Wrapped Properties¶ ラップされたプロパティに初期値を設定する¶
The code in the examples above sets the initial value for the wrapped property by giving number
an initial value in the definition of TwelveOrLess
. Code that uses this property wrapper can’t specify a different initial value for a property that’s wrapped by TwelveOrLess
—for example, the definition of SmallRectangle
can’t give height
or width
initial values. To support setting an initial value or other customization, the property wrapper needs to add an initializer. Here’s an expanded version of TwelveOrLess
called SmallNumber
that defines initializers that set the wrapped and maximum value:
上の例におけるコードは、ラップされたプロパティに初期値を設定することを、number
を初期値に与えることによって、TwelveOrLess
の定義の中で、行います。このプロパティラッパーを使うコードは、TwelveOrLess
によってラップされるプロパティに対して異なる初期値を指定できません — 例えば、SmallRectangle
の定義はheight
やwidth
に初期値を与えることができません。初期値の設定または他のカスタマイゼーションをサポートするには、プロパティラッパーはイニシャライザの追加を必要とします。ここにある拡張されたバージョンのTwelveOrLess
、SmallNumber
と呼ばれるものがあります、それはラップされたそして最大限の値を設定するイニシャライザそれらを定義します:
- @propertyWrapper
- struct SmallNumber {
- private var maximum: Int
- private var number: Int
- var wrappedValue: Int {
- get { return number }
- set { number = min(newValue, maximum) }
- }
- init() {
- maximum = 12
- number = 0
- }
- init(wrappedValue: Int) {
- maximum = 12
- number = min(wrappedValue, maximum)
- }
- init(wrappedValue: Int, maximum: Int) {
- self.maximum = maximum
- number = min(wrappedValue, maximum)
- }
- }
The definition of SmallNumber
includes three initializers—init()
, init(wrappedValue:)
, and init(wrappedValue:maximum:)
—which the examples below use to set the wrapped value and the maximum value. For information about initialization and initializer syntax, see Initialization.
SmallNumber
の定義は3つのイニシャライザを含みます — init()
、init(wrappedValue:)
、そしてinit(wrappedValue:maximum:)
— それらは、下の例がラップ値と最大値を設定するのに使用します。初期化とイニシャライザ構文についての情報として、初期化を見てください。
When you apply a wrapper to a property and you don’t specify an initial value, Swift uses the init()
initializer to set up the wrapper. For example:
あなたがラッパーをプロパティに適用するそしてあなたが初期値を指定しないならば、スウィフトはinit()
イニシャライザを使ってラッパーを準備します。例えば:
- struct ZeroRectangle {
- @SmallNumber var height: Int
- @SmallNumber var width: Int
- }
- var zeroRectangle = ZeroRectangle()
- print(zeroRectangle.height, zeroRectangle.width)
- // Prints "0 0"
The instances of SmallNumber
that wrap height
and width
are created by calling SmallNumber()
. The code inside that initializer sets the initial wrapped value and the initial maximum value, using the default values of zero and 12. The property wrapper still provides all of the initial values, like the earlier example that used TwelveOrLess
in SmallRectangle
. Unlike that example, SmallNumber
also supports writing those initial values as part of declaring the property.
height
とwidth
をラップするSmallNumber
のインスタンスは、SmallNumber()
を呼び出すことによって作成されます。そのイニシャライザの内側のコードは、初期ラップ値と初期最大値を設定します、ゼロと12の省略時の値を使って。プロパティラッパーは、依然として初期値のすべてを提供します、SmallRectangle
でのTwelveOrLess
を使った前の例のように。その例とは違い、SmallNumber
は、それら初期値を書き込むこともプロパティの宣言の一部としてサポートします。
When you specify an initial value for the property, Swift uses the init(wrappedValue:)
initializer to set up the wrapper. For example:
あなたが初期値をプロパティに対して指定する場合、スウィフトはinit(wrappedValue:)
イニシャライザを使ってラッパーを準備します。例えば:
- struct UnitRectangle {
- @SmallNumber var height: Int = 1
- @SmallNumber var width: Int = 1
- }
- var unitRectangle = UnitRectangle()
- print(unitRectangle.height, unitRectangle.width)
- // Prints "1 1"
When you write = 1
on a property with a wrapper, that’s translated into a call to the init(wrappedValue:)
initializer. The instances of SmallNumber
that wrap height
and width
are created by calling SmallNumber(wrappedValue: 1)
. The initializer uses the wrapped value that’s specified here, and it uses the default maximum value of 12.
あなたが= 1
をラッパーをもつプロパティ上で記述する場合、それはinit(wrappedValue:)
イニシャライザへの呼び出しに変換されます。height
とwidth
をラップするSmallNumber
のインスタンスは、SmallNumber(wrappedValue: 1)
を呼び出すことによって作成されます。イニシャライザは、ここで指定されるラップ値を使います、そしてそれは省略時の最大値限の12を使います。
When you write arguments in parentheses after the custom attribute, Swift uses the initializer that accepts those arguments to set up the wrapper. For example, if you provide an initial value and a maximum value, Swift uses the init(wrappedValue:maximum:)
initializer:
あなたが引数を丸括弧の中であつらえの属性の後に書く場合、スウィフトはそれらの引数を受け入れるイニシャライザを使ってそのラッパーを準備します。例えば、あなたが初期値と最大値を提供するならば、スウィフトはinit(wrappedValue:maximum:)
イニシャライザを使います:
- struct NarrowRectangle {
- @SmallNumber(wrappedValue: 2, maximum: 5) var height: Int
- @SmallNumber(wrappedValue: 3, maximum: 4) var width: Int
- }
- var narrowRectangle = NarrowRectangle()
- print(narrowRectangle.height, narrowRectangle.width)
- // Prints "2 3"
- narrowRectangle.height = 100
- narrowRectangle.width = 100
- print(narrowRectangle.height, narrowRectangle.width)
- // Prints "5 4"
The instance of SmallNumber
that wraps height
is created by calling SmallNumber(wrappedValue: 2, maximum: 5)
, and the instance that wraps width
is created by calling SmallNumber(wrappedValue: 3, maximum: 4)
.
height
をラップするSmallNumber
インスタンスは、SmallNumber(wrappedValue: 2, maximum: 5)
を呼び出すことによって作成されます、width
をラップするインスタンスは、SmallNumber(wrappedValue: 3, maximum: 4)
を呼び出すことによって作成されます。
By including arguments to the property wrapper, you can set up the initial state in the wrapper or pass other options to the wrapper when it’s created. This syntax is the most general way to use a property wrapper. You can provide whatever arguments you need to the attribute, and they’re passed to the initializer. 引数をプロパティラッパーに含めることによって、あなたは初期状態をラッパーにおいて準備することまたは他のオプションをラッパーに渡すことが、それが作成される時に可能です。この構文は、プロバティラッパーを使う最も一般的な方法です。あなたは、どんなものでもあなたが必要とする引数それらを属性に提供できます、そしてそれらはイニシャライザに渡されます。
When you include property wrapper arguments, you can also specify an initial value using assignment. Swift treats the assignment like a wrappedValue
argument and uses the initializer that accepts the arguments you include. For example:
あなたがプロパティラッパー引数を含める場合、あなたはまた代入を使って初期値を指定できます。スウィフトは、その代入をwrappedValue
引数のように扱います、そしてあなたが含める引数を受け入れるイニシャライザを使います。例えば:
- struct MixedRectangle {
- @SmallNumber var height: Int = 1
- @SmallNumber(maximum: 9) var width: Int = 2
- }
- var mixedRectangle = MixedRectangle()
- print(mixedRectangle.height)
- // Prints "1"
- mixedRectangle.height = 20
- print(mixedRectangle.height)
- // Prints "12"(「12」を出力します)
The instance of SmallNumber
that wraps height
is created by calling SmallNumber(wrappedValue: 1)
, which uses the default maximum value of 12. The instance that wraps width
is created by calling SmallNumber(wrappedValue: 2, maximum: 9)
.
height
をラップするSmallNumber
のインスタンスは、SmallNumber(wrappedValue: 1)
を呼び出すことによって作成されます、それは省略時の最大値の12を使います。width
をラップするインスタンスは、SmallNumber(wrappedValue: 2, maximum: 9)
を呼び出すことによって作成されます。
Projecting a Value From a Property Wrapper¶ 値をプロパティラッパーから投影する¶
In addition to the wrapped value, a property wrapper can expose additional functionality by defining a projected value—for example, a property wrapper that manages access to a database can expose a flushDatabaseConnection()
method on its projected value. The name of the projected value is the same as the wrapped value, except it begins with a dollar sign ($
). Because your code can’t define properties that start with $
the projected value never interferes with properties you define.
そのラップされた値に加えて、プロパティラッパーは投影値を定義することによっていっそうの機能性を露出できます — 例えば、データベースへのアクセスを管理するプロパティラッパーは、flushDatabaseConnection()
メソッドをそれの投影値上で露出できます。投影値の名前は、ラップ値と同じです、それがドル記号($
)で始まることを除いては。あなたのコードが$
で始まるプロパティを定義できないことから投影値は決してあなたが定義するプロパティと干渉しません。
In the SmallNumber
example above, if you try to set the property to a number that’s too large, the property wrapper adjusts the number before storing it. The code below adds a projectedValue
property to the SmallNumber
structure to keep track of whether the property wrapper adjusted the new value for the property before storing that new value.
上のSmallNumber
例において、あなたがプロパティをある大きすぎる数へと設定しようとするならば、プロパティラッパーはその数を調節します、それを格納する前に。下のコードは、projectedValue
プロパティをSmallNumber
構造体に加えることで、プロパティラッパーがプロパティに対する新しい値を、その新しい値を格納する前に調節したかどうかを追跡します。
- @propertyWrapper
- struct SmallNumber {
- private var number: Int
- private(set) var projectedValue: Bool
- var wrappedValue: Int {
- get { return number }
- set {
- if newValue > 12 {
- number = 12
- projectedValue = true
- } else {
- number = newValue
- projectedValue = false
- }
- }
- }
- init() {
- self.number = 0
- self.projectedValue = false
- }
- }
- struct SomeStructure {
- @SmallNumber var someNumber: Int
- }
- var someStructure = SomeStructure()
- someStructure.someNumber = 4
- print(someStructure.$someNumber)
- // Prints "false"(「false」を出力します)
- someStructure.someNumber = 55
- print(someStructure.$someNumber)
- // Prints "true"(「true」を出力します)
Writing someStructure.$someNumber
accesses the wrapper’s projected value. After storing a small number like four, the value of someStructure.$someNumber
is false
. However, the projected value is true
after trying to store a number that’s too large, like 55.
someStructure.$someNumber
と書くことは、ラッパーのもつ投影値にアクセスします。4のような小さい値を格納後、someStructure.$someNumber
の値はfalse
です。しかしながら、その投影値は、55のような大きすぎる数を格納しようとする後はtrue
です。
A property wrapper can return a value of any type as its projected value. In this example, the property wrapper exposes only one piece of information—whether the number was adjusted—so it exposes that Boolean value as its projected value. A wrapper that needs to expose more information can return an instance of some other data type, or it can return self
to expose the instance of the wrapper as its projected value.
プロパティラッパーは、あらゆる型の値をそれの投影値として返せます。この例では、プロパティラッパーは情報の一片だけを露出します — その数が調節されたかどうか — それでそれはそのブール値をそれの投影値として露出します。さらに情報を露出する必要があるラッパーは、何らかの他のデータ型のインスタンスを返せます、またはそれは、self
を返すことでラッパーのインスタンスをそれの投影値として露出できます。
When you access a projected value from code that’s part of the type, like a property getter or an instance method, you can omit self.
before the property name, just like accessing other properties. The code in the following example refers to the projected value of the wrapper around height
and width
as $height
and $width
:
あなたがある投影値に、プロパティゲッターやインスタンスメソッドのようなその型の一部であるコードからアクセスする場合、あなたはself.
をプロパティ名の前で省略できます、ちょうど他のプロパティにアクセスするように。次の例におけるコードは、height
とwidth
を囲んでいるラッパーの投影値を、$height
と$width
として参照します:
- enum Size {
- case small, large
- }
- struct SizedRectangle {
- @SmallNumber var height: Int
- @SmallNumber var width: Int
- mutating func resize(to size: Size) -> Bool {
- switch size {
- case .small:
- height = 10
- width = 20
- case .large:
- height = 100
- width = 100
- }
- return $height || $width
- }
- }
Because property wrapper syntax is just syntactic sugar for a property with a getter and a setter, accessing height
and width
behaves the same as accessing any other property. For example, the code in resize(to:)
accesses height
and width
using their property wrapper. If you call resize(to: .large)
, the switch case for .large
sets the rectangle’s height and width to 100. The wrapper prevents the value of those properties from being larger than 12, and it sets the projected value to true
, to record the fact that it adjusted their values. At the end of resize(to:)
, the return statement checks $height
and $width
to determine whether the property wrapper adjusted either height
or width
.
プロパティラッパー構文がゲッターとセッターをもつプロパティに対する単なる糖衣構文であることから、height
とwidth
にアクセスすることは、何か他のプロパティと同じ挙動をします。例えば、resize(to:)
でのコードは、height
とwidth
にそれらのプロパティラッパーを使ってアクセスします。あなたがresize(to: .large)
を呼び出すならば、.large
に対するスイッチケース節は、矩形のもつ高さと幅を100に設定します。ラッパーは、それらプロパティの値が12より大きくなることを防ぎます、そしてそれは投影値をtrue
に設定します、それがそれらの値を調節したという事実を記録するために。resize(to:)
の終わりで、return文は$height
と$width
を確認して、プロパティラッパーがheight
またはwidth
のどちらを調節したかを決定します。
Global and Local Variables¶ グローバルおよびローカル変数¶
The capabilities described above for computing and observing properties are also available to global variables and local variables. Global variables are variables that are defined outside of any function, method, closure, or type context. Local variables are variables that are defined within a function, method, or closure context. 計算するそして監視するプロパティに対して上で記述される可能なことは、また、グローバル変数とローカル変数に対して利用できます。グローバルな変数は、あらゆる関数、メソッド、クロージャ、または型の文脈の外で定義される変数です。ローカル変数は、関数、メソッド、またはクロージャの文脈の範囲内で定義される変数です。
The global and local variables you have encountered in previous chapters have all been stored variables. Stored variables, like stored properties, provide storage for a value of a certain type and allow that value to be set and retrieved. あなたが前の章において遭遇したグローバルおよびローカル変数は、すべて格納変数でした。格納変数は、格納プロパティの様に、保管場所を特定の型のひとつの値のために用意して、その値の設定と取得を可能にします。
However, you can also define computed variables and define observers for stored variables, in either a global or local scope. Computed variables calculate their value, rather than storing it, and they’re written in the same way as computed properties. しかし、あなたはまた、計算変数を定義すること、そして格納変数のためにオブザーバーを定義することが、グローバルなまたはローカルなスコープのどちらにおいても可能です。計算変数は値を計算します、それの貯蔵ではなくて、そして計算プロパティと同じ方法で書かれます。
Note 注意
Global constants and variables are always computed lazily, in a similar manner to Lazy Stored Properties. Unlike lazy stored properties, global constants and variables don’t need to be marked with the lazy
modifier.
グローバルな定数と変数は、遅延格納プロパティと似たやり方で、常に遅延計算されます。遅延格納プロパティと違って、グローバルな定数と変数はlazy
修飾子で印付けされる必要がありません。
Local constants and variables are never computed lazily. ローカルな定数と変数は、決して遅延して計算されません。
You can apply a property wrapper to a local stored variable, but not to a global variable or a computed variable. For example, in the code below, myNumber
uses SmallNumber
as a property wrapper.
あなたは、プロパティラッパーをローカル格納変数に適用できます、しかしグローパル変数または計算変数にはできません。例えば、下のコードにおいて、myNumber
はSmallNumber
をプロパティラッパーとして使います。
- func someFunction() {
- @SmallNumber var myNumber: Int = 0
- myNumber = 10
- // now myNumber is 10
- myNumber = 24
- // now myNumber is 12
- }
Like when you apply SmallNumber
to a property, setting the value of myNumber
to 10 is valid. Because the property wrapper doesn’t allow values higher than 12, it sets myNumber
to 12 instead of 24.
あなたがSmallNumber
に適用する時のように、myNumber
の値を10に設定することは有効です。プロパティラッパーが12より高い値を許可しないので、それはmyNumber
を12に設定します、24の代わりに。
Type Properties¶ 型プロパティ¶
Instance properties are properties that belong to an instance of a particular type. Every time you create a new instance of that type, it has its own set of property values, separate from any other instance. インスタンスプロパティは、ある特定の型のあるひとつのインスタンスに属しているプロパティです。あなたがその型の新しいインスタンスをつくるたびに、それは、他のあらゆるインスタンスから独立した、独自のひと組のプロパティ値を持ちます。
You can also define properties that belong to the type itself, not to any one instance of that type. There will only ever be one copy of these properties, no matter how many instances of that type you create. These kinds of properties are called type properties. あなたは、また、その型のどれかひとつのインスタンスにではなく、型それ自体に属しているいくらかのプロパティを定義することができます。それらのプロパティのコピーはただ1つだけ存在することになります、あなたが作るその型のインスタンスがいくらあろうとも。これらの種類のプロパティは、型プロパティと呼ばれています。
Type properties are useful for defining values that are universal to all instances of a particular type, such as a constant property that all instances can use (like a static constant in C), or a variable property that stores a value that’s global to all instances of that type (like a static variable in C). 型プロパティは、特定の型の全てのインスタンスに共通なさまざまな値を定義することに役立ちます、例えば、全てのインスタンスが使うことができる定数プロパティ(Cでの静的定数のように)、またはその型の全てのインスタンスにグローバルである値を格納する変数プロパティ(Cでの静的変数のように)。
Stored type properties can be variables or constants. Computed type properties are always declared as variable properties, in the same way as computed instance properties. 格納プロパティは、変数または定数であることができます。計算型プロパティは、計算インスタンスプロパティと同じ方法で、常に変数プロパティとして宣言されます。
Note 注意
Unlike stored instance properties, you must always give stored type properties a default value. This is because the type itself doesn’t have an initializer that can assign a value to a stored type property at initialization time. 格納インスタンスプロパティと違って、あなたは格納型プロパティに省略時の値を常に与えなければなりません。これは、型それ自身には初期化時に値を格納型プロパティに代入することができるイニシャライザがないからです。
Stored type properties are lazily initialized on their first access. They’re guaranteed to be initialized only once, even when accessed by multiple threads simultaneously, and they don’t need to be marked with the lazy
modifier.
格納型プロパティは、それらの最初のアクセスにおいて遅延して初期化されます。それらは、ただ一度だけ初期化されることを保証されます、複数のスレッドによって同時にアクセスされる場合でさえもです、そしてそれらはlazy
修飾子で印されることを必要としません。
Type Property Syntax¶ 型プロパティ構文¶
In C and Objective-C, you define static constants and variables associated with a type as global static variables. In Swift, however, type properties are written as part of the type’s definition, within the type’s outer curly braces, and each type property is explicitly scoped to the type it supports. CとObjective-Cでは、あなたは静的定数およびグローバルな静的変数として型と結びついた変数を定義します。スウィフトでは、しかしながら、型プロパティは、型の定義の一部として、型の外縁の波括弧の内部に書かれます、そして各型プロパティは、明確にそれが支持する型にスコープを定められます。
You define type properties with the static
keyword. For computed type properties for class types, you can use the class
keyword instead to allow subclasses to override the superclass’s implementation. The example below shows the syntax for stored and computed type properties:
あなたは、型プロパティをstatic
キーワードを使って定義します。クラス型のための計算型プロパティに対しては、あなたは代わりにclass
キーワードを使って、サブクラスにそのスーパークラスの実装のオーバーライドを許可することができます。以下の例は、格納型プロパティおよび計算型プロパティのための構文を示します:
- struct SomeStructure {
- static var storedTypeProperty = "Some value."
- static var computedTypeProperty: Int {
- return 1
- }
- }
- enum SomeEnumeration {
- static var storedTypeProperty = "Some value."
- static var computedTypeProperty: Int {
- return 6
- }
- }
- class SomeClass {
- static var storedTypeProperty = "Some value."
- static var computedTypeProperty: Int {
- return 27
- }
- class var overrideableComputedTypeProperty: Int {
- return 107
- }
- }
Note 注意
The computed type property examples above are for read-only computed type properties, but you can also define read-write computed type properties with the same syntax as for computed instance properties. 上の計算型プロパティの例は、読み出し専用の計算型プロパティのためのものです、しかしあなたはまた、計算インスタンスプロパティについては同じ構文をつかって読み書き両用の計算型プロパティを定義することができます。
Querying and Setting Type Properties¶ 型プロパティを問い合わせて、設定する¶
Type properties are queried and set with dot syntax, just like instance properties. However, type properties are queried and set on the type, not on an instance of that type. For example: 型プロパティは、インスタンスプロパティのように、ドット構文で問い合わせられ設定されます。しかし、型プロパティは、その型のインスタンスにではなく、その型に問い合わせられ設定されます。例えば:
- print(SomeStructure.storedTypeProperty)
- // Prints "Some value."(「ある値。」を出力します)
- SomeStructure.storedTypeProperty = "Another value."
- print(SomeStructure.storedTypeProperty)
- // Prints "Another value."(「別の値。」を出力します)
- print(SomeEnumeration.computedTypeProperty)
- // Prints "6"(「6」を出力します)
- print(SomeClass.computedTypeProperty)
- // Prints "27"(「27」を出力します)
The examples that follow use two stored type properties as part of a structure that models an audio level meter for a number of audio channels. Each channel has an integer audio level between 0
and 10
inclusive.
あとに続く例は、いくつかのオーディオ・チャンネル用のオーディオ・レベルをモデル化する構造体の一部として、2つの格納型プロパティを使います。各チャンネルは、0
から10
を含むまでの整数オーディオ・レベルがあります。
The figure below illustrates how two of these audio channels can be combined to model a stereo audio level meter. When a channel’s audio level is 0
, none of the lights for that channel are lit. When the audio level is 10
, all of the lights for that channel are lit. In this figure, the left channel has a current level of 9
, and the right channel has a current level of 7
:
下の図は、これらの音声のチャンネルのうちの2つがステレオ音声のレベル・メーターをモデル化するために結合されることができる方法を図示します。チャンネルの音声のレベルが0
であるとき、そのチャンネルのための光のどれも点灯されません。音声のレベルが10
であるとき、そのチャンネルのための光の全ては点灯されます。この図には、左のチャンネルに現在9
のレベルがあり、右のチャンネルに現在7
のレベルがあります:
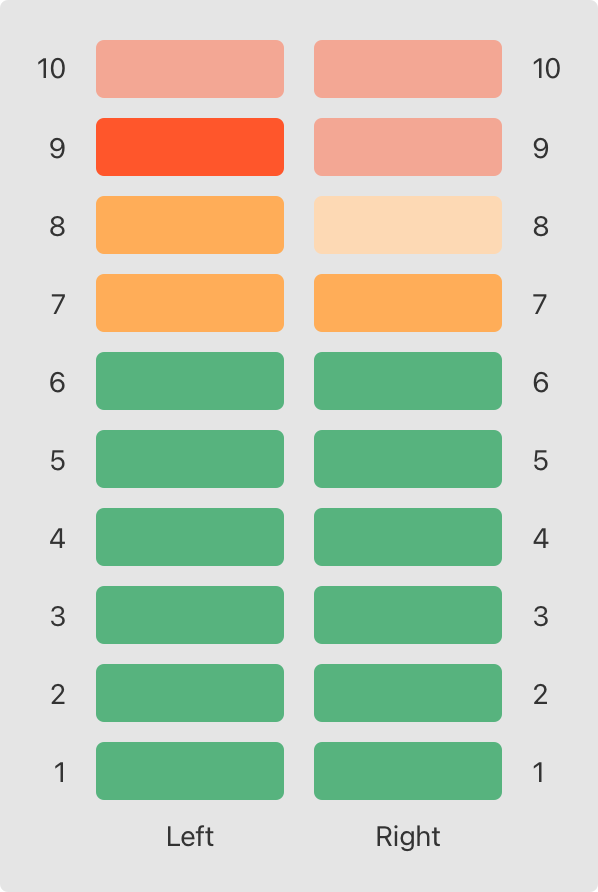
The audio channels described above are represented by instances of the AudioChannel
structure:
上で記述される音声のチャンネルは、AudioChannel
構造体のインスタンスによって表されます:
- struct AudioChannel {
- static let thresholdLevel = 10
- static var maxInputLevelForAllChannels = 0
- var currentLevel: Int = 0 {
- didSet {
- if currentLevel > AudioChannel.thresholdLevel {
- // cap the new audio level to the threshold level(新しい音声レベルを限界レベルに制限する)
- currentLevel = AudioChannel.thresholdLevel
- }
- if currentLevel > AudioChannel.maxInputLevelForAllChannels {
- // store this as the new overall maximum input level(これを新しい全体で最大の入力レベルとして格納する)
- AudioChannel.maxInputLevelForAllChannels = currentLevel
- }
- }
- }
- }
The AudioChannel
structure defines two stored type properties to support its functionality. The first, thresholdLevel
, defines the maximum threshold value an audio level can take. This is a constant value of 10
for all AudioChannel
instances. If an audio signal comes in with a higher value than 10
, it will be capped to this threshold value (as described below).
AudioChannel
構造体は、その機能性を支えるために2つの格納型プロパティを定義します。その一番目、thresholdLevel
は、音声レベルがとることができる最大の限界値を定義します。これは、定数値で、全てのAudioChannel
インスタンスのために10
です。ある音声信号が10
より高い値でやってくるならば、それはこの限界値に制限されます(下記のように)。
The second type property is a variable stored property called maxInputLevelForAllChannels
. This keeps track of the maximum input value that has been received by any AudioChannel
instance. It starts with an initial value of 0
.
第二の型プロパティは、maxInputLevelForAllChannels
と呼ばれる変数格納プロパティです。これは、あらゆる AudioChannel
インスタンスによって受け取られた最大入力値の情報を得続けます。それは、初期値の0
から始めます。
The AudioChannel
structure also defines a stored instance property called currentLevel
, which represents the channel’s current audio level on a scale of 0
to 10
.
AudioChannel
構造体はまた、currentLevel
と呼ばれる格納インスタンスプロパティを定義します、それは、0
から10
までの目盛りでチャンネルの現在の音声レベルを表わします。
The currentLevel
property has a didSet
property observer to check the value of currentLevel
whenever it’s set. This observer performs two checks:
currentLevel
プロパティはdidSet
プロパティオブザーバーを持ち、currentLevel
の値をそれが設定されるときはいつでも調べるようにします。このオブザーバーは、2つのチェックをします:
- If the new value of
currentLevel
is greater than the allowedthresholdLevel
, the property observer capscurrentLevel
tothresholdLevel
.currentLevel
の新しい値が許可されたthresholdLevel
より大きいならば、このプロパティオブザーバーはcurrentLevel
をthresholdLevel
に制限します。 - If the new value of
currentLevel
(after any capping) is higher than any value previously received by anyAudioChannel
instance, the property observer stores the newcurrentLevel
value in themaxInputLevelForAllChannels
type property.currentLevel
の新しい値が(制限を受けた後に)あらゆるAudioChannel
インスタンスで以前に受けた値より高いならば、プロパティオブザーバーは、新しいcurrentLevel
値を型プロパティmaxInputLevelForAllChannels
に保管します。
Note 注意
In the first of these two checks, the didSet
observer sets currentLevel
to a different value. This doesn’t, however, cause the observer to be called again.
これらの2つのチェックで最初のものにおいて、didSet
オブザーバーは、currentLevel
を異なる値に設定します。これは、しかし、このオブザーバーが再び呼び出されるようにしません。
You can use the AudioChannel
structure to create two new audio channels called leftChannel
and rightChannel
, to represent the audio levels of a stereo sound system:
あなたは、AudioChannel
構造体を、leftChannel
とrightChannel
と呼ばれる2つの新しい音声チャンネルをつくるために使用して、ステレオ・オーディオ・システムの音声のレベルを表すことができます:
- var leftChannel = AudioChannel()
- var rightChannel = AudioChannel()
If you set the currentLevel
of the left channel to 7
, you can see that the maxInputLevelForAllChannels
type property is updated to equal 7
:
あなたが左のチャンネルのcurrentLevel
を7
に設定するならば、あなたは型プロパティmaxInputLevelForAllChannels
が7
に等しくなるよう更新されるのを見ることができます:
- leftChannel.currentLevel = 7
- print(leftChannel.currentLevel)
- // Prints "7"(「7」を出力します)
- print(AudioChannel.maxInputLevelForAllChannels)
- // Prints "7"(「7」を出力します)
If you try to set the currentLevel
of the right channel to 11
, you can see that the right channel’s currentLevel
property is capped to the maximum value of 10
, and the maxInputLevelForAllChannels
type property is updated to equal 10
:
あなたが右のチャンネルのcurrentLevel
を11
に設定しようとするならば、あなたは右のチャンネルのcurrentLevel
プロパティが最大値の10
に制限されるのを見ることができます、そして型プロパティmaxInputLevelForAllChannels
は10
に等しくなるよう更新されます:
- rightChannel.currentLevel = 11
- print(rightChannel.currentLevel)
- // Prints "10"(「10」を出力します)
- print(AudioChannel.maxInputLevelForAllChannels)
- // Prints "10"(「10」を出力します)