Strings and Characters¶ 文字列と文字¶
A string is a series of characters, such as "hello, world"
or "albatross"
. Swift strings are represented by the String
type. The contents of a String
can be accessed in various ways, including as a collection of Character
values.
ひとつの文字列は、一連なりの文字です、例えば"hello, world"
または"albatross"
など。スウィフトの文字列はString
型によって表されます。あるString
の内容は、いくつかのやり方でアクセスされることができます、それにはCharacter
値からなるひとつのコレクションとしてを含みます。
Swift’s String
and Character
types provide a fast, Unicode-compliant way to work with text in your code. The syntax for string creation and manipulation is lightweight and readable, with a string literal syntax that’s similar to C. String concatenation is as simple as combining two strings with the +
operator, and string mutability is managed by choosing between a constant or a variable, just like any other value in Swift. You can also use strings to insert constants, variables, literals, and expressions into longer strings, in a process known as string interpolation. This makes it easy to create custom string values for display, storage, and printing.
スウィフトのString
およびCharacter
型は、あなたのコードにおいてテキストを処理するために、ある高速な、Unicodeに対応したやり方を提供します。文字列の作成と操作のための構文は、軽量で、読みやすく、Cに類似した文字列リテラル構文をもちます。文字列の連結は、2つの文字列を+
演算子を使って繋ぎ合わせるくらい単純です、そして、文字列が変更可能かどうかは、スウィフトの他あらゆる値と同じように、定数または変数のどちらかを選ぶことによって管理されます。あなたはまた文字列を、文字列補間として知られているやり方で、定数、変数、リテラル、および式をより長い文字列に差し入れるために使うことができます。これは、表示、保存、そして出力するために個々の注文に応じた文字列値をつくることを簡単にします。
Despite this simplicity of syntax, Swift’s String
type is a fast, modern string implementation. Every string is composed of encoding-independent Unicode characters, and provides support for accessing those characters in various Unicode representations.
構文のこの単純さにもかかわらず、スウィフトのString
型は、速く、現代的な文字列の実装です。あらゆる文字列は、符号化に依存しないUnicode文字から構成されます、そして多彩なUnicode表現においてそのような文字にアクセスするための支援を提供します。
Note 注意
Swift’s String
type is bridged with Foundation’s NSString
class. Foundation also extends String
to expose methods defined by NSString
. This means, if you import Foundation, you can access those NSString
methods on String
without casting.
スウィフトのString
型は、Foundation
のNSStringクラスを使って橋渡しをされます。Foundationはまた、String
を拡張してNSString
によって定義されるメソッドに触れさせます。これが意味するのは、あなたがFoundationをインポートするならば、あなたはそれらNSString
メソッドにString
上でキャストなしでアクセスできるという事です。
For more information about using String
with Foundation and Cocoa, see Bridging Between String and NSString.
FoundationとCocoaとともにString
を使うことについてのさらなる情報として、StringとNSStringの間のブリッジを見てください。
String Literals¶ 文字列リテラル¶
You can include predefined String
values within your code as string literals. A string literal is a sequence of characters surrounded by double quotation marks ("
).
あなたは、あらかじめ定義されたString
値を文字列リテラルとしてあなたのコードの内部に含めることができます。文字列リテラルは、二重引用符("
)によって囲まれた一連の文字です。
Use a string literal as an initial value for a constant or variable: 文字列リテラルを定数または変数の初期値として使ってください:
- let someString = "Some string literal value"
Note that Swift infers a type of String
for the someString
constant because it’s initialized with a string literal value.
スウィフトが型String
をsomeString
定数に対して推論することに注意してください、それが文字列リテラル値で初期化されるためです。
Multiline String Literals¶ 複数行文字列リテラル¶
If you need a string that spans several lines, use a multiline string literal—a sequence of characters surrounded by three double quotation marks: あなたがいくつかの行に及ぶ文字列を必要とするならば、複数行文字列リテラル — 3つの二重引用符で囲まれる一連の文字、を使ってください:
- let quotation = """
- The White Rabbit put on his spectacles. "Where shall I begin,
- please your Majesty?" he asked.
- "Begin at the beginning," the King said gravely, "and go on
- till you come to the end; then stop."
- """
A multiline string literal includes all of the lines between its opening and closing quotation marks. The string begins on the first line after the opening quotation marks ("""
) and ends on the line before the closing quotation marks, which means that neither of the strings below start or end with a line break:
複数行文字列リテラルは、それの開始および終了引用符の間のすべての行を含みます。文字列は、開始引用符("""
)の後の最初の行で始まって終了引用符の前の行で終わります、それが意味するのは下の文字列のどちらも改行で始まったり終わったりしないということです。
- let singleLineString = "These are the same."
- let multilineString = """
- These are the same.
- """
When your source code includes a line break inside of a multiline string literal, that line break also appears in the string’s value. If you want to use line breaks to make your source code easier to read, but you don’t want the line breaks to be part of the string’s value, write a backslash (\
) at the end of those lines:
あなたのソースコードがある複数行文字列リテラルの内部に改行を含む場合、その改行はまたその文字列の持つ値の中に現れます。あなたが改行を使うことであなたのソースコードを読みやすくしたい、しかしあなたがその改行に文字列の値の一部であることを望まないならば、バックスラッシュを(\
)それらの行の終わりに書いてください:
- let softWrappedQuotation = """
- The White Rabbit put on his spectacles. "Where shall I begin, \
- please your Majesty?" he asked.
- "Begin at the beginning," the King said gravely, "and go on \
- till you come to the end; then stop."
- """
To make a multiline string literal that begins or ends with a line feed, write a blank line as the first or last line. For example: ラインフィードで始まるまたは終わる複数行文字列リテラルを作るには、空の行を最初または最後の行として記述します。例えば:
- let lineBreaks = """
- This string starts with a line break.
- It also ends with a line break.
- """
A multiline string can be indented to match the surrounding code. The whitespace before the closing quotation marks ("""
) tells Swift what whitespace to ignore before all of the other lines. However, if you write whitespace at the beginning of a line in addition to what’s before the closing quotation marks, that whitespace is included.
複数行文字列は、字下げされることによって、囲んでいるコードに調和します。終了引用符("""
)の前の空白文字は、他の行すべての前でどの空白文字を無視するかスウィフトに教えます。しかしながら、あなたが終了引用符の前のものに加えて空白文字をある行の始まりで書くならば、その空白文字は含められます。
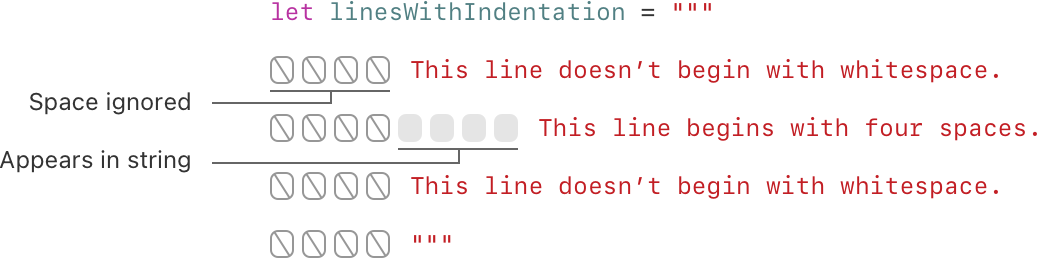
In the example above, even though the entire multiline string literal is indented, the first and last lines in the string don’t begin with any whitespace. The middle line has more indentation than the closing quotation marks, so it starts with that extra four-space indentation. 上の例において、たとえ複数行リテラル全体が字下げされるとしても、その文字列の最初と最後の行は全く空白で始まりません。真ん中の行は終了引用符よりもっと字下げされます、なのでそれは追加の4つの空白の字下げで始まります。
Special Characters in String Literals¶ 文字列リテラル内の特別な文字¶
String literals can include the following special characters: 文字列リテラルは、以下の特別な文字を含むことができます:
- The escaped special characters
\0
(null character),\\
(backslash),\t
(horizontal tab),\n
(line feed),\r
(carriage return),\"
(double quotation mark) and\'
(single quotation mark) エスケープされた特殊文字の\0
(ヌル文字)、\\
(バックスラッシュ)、\t
(水平タブ)、\n
(ラインフィード)、\r
(キャリッジリターン)、\"
(二重引用符)と\'
(一重引用符) - An arbitrary Unicode scalar value, written as
\u{
n}
, where n is a 1–8 digit hexadecimal number (Unicode is discussed in Unicode below) 随意のユニコードスカラー値、\u{
n}
のように書かれます、ここでnは1–8桁の16進数です(ユニコードは下のUnicodeで議論されます)
The code below shows four examples of these special characters. The wiseWords
constant contains two escaped double quotation marks. The dollarSign
, blackHeart
, and sparklingHeart
constants demonstrate the Unicode scalar format:
以下のコードは、これらの特殊文字の4つの例を示します。定数のwiseWords
は、2つのエスケープされた二重引用符文字を含みます。dollarSign
、blackHeart
、そしてsparklingHeart
定数は、Unicodeスカラーの書式を示します:
- let wiseWords = "\"Imagination is more important than knowledge\" - Einstein"
- // "Imagination is more important than knowledge" - Einstein(「想像は知識より重要です」 - アインシュタイン)
- let dollarSign = "\u{24}" // $, Unicode scalar U+0024
- let blackHeart = "\u{2665}" // ♥, Unicode scalar U+2665
- let sparklingHeart = "\u{1F496}" // 💖, Unicode scalar U+1F496
Because multiline string literals use three double quotation marks instead of just one, you can include a double quotation mark ("
) inside of a multiline string literal without escaping it. To include the text """
in a multiline string, escape at least one of the quotation marks. For example:
複数行文字列リテラルは、ただ1つではなく3つの二重引用符を使います、あなたは二重引用符("
)を複数行文字列リテラルの内部にそれをエスケープすることなく含めることができます。テキスト"""
を複数行文字列の中に含めるには、少なくとも1つの引用符記号の1つをエスケープしてください。例えば:
- let threeDoubleQuotationMarks = """
- Escaping the first quotation mark \"""
- Escaping all three quotation marks \"\"\"
- """
Extended String Delimiters¶ 拡張文字列区切り記号¶
You can place a string literal within extended delimiters to include special characters in a string without invoking their effect. You place your string within quotation marks ("
) and surround that with number signs (#
). For example, printing the string literal #"Line 1\nLine 2"#
prints the line feed escape sequence (\n
) rather than printing the string across two lines.
あなたは、文字列リテラルを拡張区切り記号内に置くことで、いくらかの特殊文字を文字列の中にそれらの効果を発動することなしに含めることができます。あなたは、あなたの文字列を引用符("
)内に置きます、そしてそれをシャープ記号(#
)で囲みます。例えば、文字列リテラル#"Line 1\nLine 2"#
をブリントすることは、行送りエスケープシーケンス(\n
)をプリントします、プリントする文字列が2つの行に渡るのではなく。
If you need the special effects of a character in a string literal, match the number of number signs within the string following the escape character (\
). For example, if your string is #"Line 1\nLine 2"#
and you want to break the line, you can use #"Line 1\#nLine 2"#
instead. Similarly, ###"Line1\###nLine2"###
also breaks the line.
あなたが文字列リテラルの中のある文字の特別な効果を必要とするならば、文字列内のエスケープ文字(\
)に続くシャープ記号の数を一致させてください。例えば、あなたの文字列が#"Line 1\nLine 2"#
であるそしてあなたが行を改めることを望むならば、あなたは#"Line 1\#nLine 2"#
を代わりに使用できます。同様に、###"Line1\###nLine2"###
もまた行を改めます。
String literals created using extended delimiters can also be multiline string literals. You can use extended delimiters to include the text """
in a multiline string, overriding the default behavior that ends the literal. For example:
拡張区切り文字を使って作成される文字列リテラルは、また複数行文字列リテラルであることができます。あなたは拡張区切り文字を使うことで、テキスト"""
を複数行文字列の中に含めることが、省略時の挙動であるリテラルの終わりをオーバーライドして行えます。例えば:
- let threeMoreDoubleQuotationMarks = #"""
- Here are three more double quotes: """
- """#
Initializing an Empty String¶ 空の文字列を初期化する¶
To create an empty String
value as the starting point for building a longer string, either assign an empty string literal to a variable, or initialize a new String
instance with initializer syntax:
より長い文字列をつくるための出発点として空のString
値を作成するために、変数に空のリテラル文字列を代入するか、イニシャライザ構文で新しいString
インスタンスを初期化してください:
- var emptyString = "" // empty string literal(空の文字列リテラル)
- var anotherEmptyString = String() // initializer syntax(イニシャライザ構文)
- // these two strings are both empty, and are equivalent to each other(これらの2つの文字列は両方とも空で、お互いに等しいです)
Find out whether a String
value is empty by checking its Boolean isEmpty
property:
あるString
値が空かどうかを、それのブールのisEmpty
プロパティを調べることで確認してください:
- if emptyString.isEmpty {
- print("Nothing to see here")
- }
- // Prints "Nothing to see here"(「ここで見るものは何も無し」を出力します)
String Mutability¶ 文字列の可変性¶
You indicate whether a particular String
can be modified (or mutated) by assigning it to a variable (in which case it can be modified), or to a constant (in which case it can’t be modified):
あなたは、特定のString
が修正される(または変化する)ことができるかどうか、それを変数(その場合それは修が可能です)にまたは定数(その場合それは修正できません)に代入することによって示します:
- var variableString = "Horse"
- variableString += " and carriage"
- // variableString is now "Horse and carriage"(variableStringは、現在「馬と馬車」です)
- let constantString = "Highlander"
- constantString += " and another Highlander"
- // this reports a compile-time error - a constant string cannot be modified(これはコンパイル時エラー - 定数文字列は修正できません、を報告します)
Note 注意
This approach is different from string mutation in Objective-C and Cocoa, where you choose between two classes (NSString
and NSMutableString
) to indicate whether a string can be mutated.
この取り組みは、Objective-Cとココアでの文字列変化と異なります、そこでは、あなたは文字列が変化することができるかどうか示すために、2つのクラス(NSString
とNSMutableString
)のどちらかを選びます。
Strings Are Value Types¶ 文字列は値型です¶
Swift’s String
type is a value type. If you create a new String
value, that String
value is copied when it’s passed to a function or method, or when it’s assigned to a constant or variable. In each case, a new copy of the existing String
value is created, and the new copy is passed or assigned, not the original version. Value types are described in Structures and Enumerations Are Value Types.
スウィフトのString
型は、値型です。あなたが新しいString
値をつくるならば、そのString
値は、それが関数またはメソッドに渡される時に、またはそれが定数または変数に代入される時に、コピーされます。それぞれの場合において、既存のString
値の新しいコピーがつくられます、そしてその新しいコピーが渡されるか代入されます、元々のものではなく。値型は「構造体と列挙は値型です」で記述されます。
Swift’s copy-by-default String
behavior ensures that when a function or method passes you a String
value, it’s clear that you own that exact String
value, regardless of where it came from. You can be confident that the string you are passed won’t be modified unless you modify it yourself.
スウィフトの省略時コピーのString
挙動は、関数またはメソッドがあなたにString
値を渡すとき、それが来たところに関係なく、あなたがその正確な写しのString
値を所有することが明白なのを確実にします。あなたは、あなたが渡される文字列があなたが自身でそれを修正しない限り修正されないことを確信することができます。
Behind the scenes, Swift’s compiler optimizes string usage so that actual copying takes place only when absolutely necessary. This means you always get great performance when working with strings as value types. 舞台裏で、スウィフトのコンパイラは、実際にコピーすることは絶対に必要なときだけ起こるように、文字列使用を最適化します。これは、値型として文字列を扱うとき、あなたは常に非常に大きいパフォーマンスを得ることを意味します。
Working with Characters¶ 文字を扱う¶
You can access the individual Character
values for a String
by iterating over the string with a for
-in
loop:
あなたは、あるString
の個々のCharacter
値にfor
-in
ループでその文字列の初めから終わりまで反復することによってアクセスできます:
- for character in "Dog!🐶" {
- print(character)
- }
- // D
- // o
- // g
- // !
- // 🐶
The for
-in
loop is described in For-In Loops.
for
-in
ループはfor-inループで記述されます。
Alternatively, you can create a stand-alone Character
constant or variable from a single-character string literal by providing a Character
type annotation:
あるいは、あなたは1文字だけの文字列リテラルから単独のCharacter
定数または変数を作成することが、Character
型注釈を提供することによって可能です:
- let exclamationMark: Character = "!"
String
values can be constructed by passing an array of Character
values as an argument to its initializer:
String
値は、Character
値の配列をそれのイニシャライザへの引数として渡すことによって組み立てられることができます。
- let catCharacters: [Character] = ["C", "a", "t", "!", "🐱"]
- let catString = String(catCharacters)
- print(catString)
- // Prints "Cat!🐱"
Concatenating Strings and Characters¶ 文字列と文字の連結¶
String
values can be added together (or concatenated) with the addition operator (+
) to create a new String
value:
String
値は、新しいString
値をつくるために加算演算子(+
)を使って1つに足し合わされる(または連結される)ことができます:
- let string1 = "hello"
- let string2 = " there"
- var welcome = string1 + string2
- // welcome now equals "hello there"(welcomeは、現在「やあ、こんにちは」に等しい)
You can also append a String
value to an existing String
variable with the addition assignment operator (+=
):
あなたは、また、あるString
値を既存のString
変数に加算代入演算子(+=
)を使って追加することができます:
- var instruction = "look over"
- instruction += string2
- // instruction now equals "look over there"(instructionは、現在「あそこを見て」に等しい)
You can append a Character
value to a String
variable with the String
type’s append()
method:
あなたは、Character
値をString
変数にそのString型
のもつappend()
メソッドを使って追加することができます:
- let exclamationMark: Character = "!"
- welcome.append(exclamationMark)
- // welcome now equals "hello there!"(welcomeは、現在「やあ!、こんにちは」に等しい)
Note 注意
You can’t append a String
or Character
to an existing Character
variable, because a Character
value must contain a single character only.
あなたはString
またはCharacter
を既存のCharacter
変数に追加することはできません、なぜならCharacter
値がただ1つの文字だけを含まなければならないためです。
If you’re using multiline string literals to build up the lines of a longer string, you want every line in the string to end with a line break, including the last line. For example: あなたが複数行文字列リテラルを使ってたくさんの行の長い文字列を作り上げようとしているならば、あなたはその文字列のすべての行を、最後の行を含めて改行で終わりたいと思うでしょう。例えば:
- let badStart = """
- one
- two
- """
- let end = """
- three
- """
- print(badStart + end)
- // Prints two lines:
- // one
- // twothree
- let goodStart = """
- one
- two
- """
- print(goodStart + end)
- // Prints three lines:
- // one
- // two
- // three
In the code above, concatenating badStart
with end
produces a two-line string, which isn’t the desired result. Because the last line of badStart
doesn’t end with a line break, that line gets combined with the first line of end
. In contrast, both lines of goodStart
end with a line break, so when it’s combined with end
the result has three lines, as expected.
上のコードにおいて、badStart
をend
と連結することは、2行の文字列を生成します、それは望んだ結果ではありません。badStart
の最後の行は改行で終わらないことから、その行はend
の最初の行と結合されます。対照的に、goodStart
の行は両方とも改行で終わります、それでそれがend
と結合される場合その結果は3行になります、予想通りに。
String Interpolation¶ 文字列補間¶
String interpolation is a way to construct a new String
value from a mix of constants, variables, literals, and expressions by including their values inside a string literal. You can use string interpolation in both single-line and multiline string literals. Each item that you insert into the string literal is wrapped in a pair of parentheses, prefixed by a backslash (\
):
文字列補間は、定数、変数、リテラル、および式の混合から、それらの値をひとつのリテラル文字列に含めることで、新しいString
値を造る方法です。あなたは、文字列補間を単一行および複数行文字列リテラルにおいて使うことができます。あなたがリテラル文字列に差し込む各項目は、一対の丸括弧に包まれて、バックスラッシュ(\
)を前に置かれます:
- let multiplier = 3
- let message = "\(multiplier) times 2.5 is \(Double(multiplier) * 2.5)"
- // message is "3 times 2.5 is 7.5"(messageは、「3かける2.5は7.5」です)
In the example above, the value of multiplier
is inserted into a string literal as \(multiplier)
. This placeholder is replaced with the actual value of multiplier
when the string interpolation is evaluated to create an actual string.
上の例で、multiplier
の値は、文字列リテラルに\(multiplier)
として差し込まれます。このプレースホルダーは、文字列補間が実際の文字列を作成するために評価されるとき、multiplier
の実際の値と取り替えられます。
The value of multiplier
is also part of a larger expression later in the string. This expression calculates the value of Double(multiplier) * 2.5
and inserts the result (7.5
) into the string. In this case, the expression is written as \(Double(multiplier) * 2.5)
when it’s included inside the string literal.
multiplier
の値は、また、文字列の後半のより大きな式の一部でもあります。この式は、Double(multiplier) * 2.5
の値を計算して、結果(7.5
)を文字列に差し込みます。この場合、式は、それが文字列リテラルに含められる時、\(Double(multiplier) * 2.5)
と書かれます。
You can use extended string delimiters to create strings containing characters that would otherwise be treated as a string interpolation. For example: あなたは、拡張文字列区切り記号を使うことで、そうしなければ文字列補間とみなされる文字を含んでいる文字列を作成できます。例えば:
- print(#"Write an interpolated string in Swift using \(multiplier)."#)
- // Prints "Write an interpolated string in Swift using \(multiplier)."
To use string interpolation inside a string that uses extended delimiters, match the number of number signs after the backslash to the number of number signs at the beginning and end of the string. For example: 文字列補間を拡張区切り記号を使う文字列内部で使うには、バックスラッシュの後のシャープ記号の数をその文字列の始まりと終わりでのシャープ記号の数と合わせてください。例えば:
- print(#"6 times 7 is \#(6 * 7)."#)
- // Prints "6 times 7 is 42."
Note 注意
The expressions you write inside parentheses within an interpolated string can’t contain an unescaped backslash (\
), a carriage return, or a line feed. However, they can contain other string literals.
補間文字列においてあなたが丸括弧内に記述する式は、エスケープされないバックスラッシュ(\
)、キャリッジリターン、またはラインフィードを含むことができません。しかしながら、それは、他の文字列リテラルを含むことができます。
Unicode¶ ユニコード¶
Unicode is an international standard for encoding, representing, and processing text in different writing systems. It enables you to represent almost any character from any language in a standardized form, and to read and write those characters to and from an external source such as a text file or web page. Swift’s String
and Character
types are fully Unicode-compliant, as described in this section.
ユニコードは、異なる表記体系のテキストを符号化、表現、そして処理するための国際的な基準です。それは、あなたにほとんどどんな文字でもどんな言語のものでも標準化された方式で表わすこと、そしてそれらの文字を、テキスト・ファイルやウェブ・ページのような外部のソースへ書き込んだり、それらから読み込んだりすることを可能にします。スウィフトのString
とCharacter
型は、この節で記述されるように、完全にUnicodeに対応しています。
Unicode Scalar Values¶ ユニコードスカラー値¶
Behind the scenes, Swift’s native String
type is built from Unicode scalar values. A Unicode scalar value is a unique 21-bit number for a character or modifier, such as U+0061
for LATIN SMALL LETTER A
("a"
), or U+1F425
for FRONT-FACING BABY CHICK
("🐥"
).
舞台裏で、スウィフト生得のString
型はユニコードスカラー値によって組み立てられています。あるユニコードスカラー値は、ある文字または修飾子のための固有な21ビットの数字です、例えばU+0061
はLATIN SMALL LETTER A
("a"
)に対して、またはU+1F425
はFRONT-FACING BABY CHICK
("🐥"
)に対してなど。
Note that not all 21-bit Unicode scalar values are assigned to a character—some scalars are reserved for future assignment or for use in UTF-16 encoding. Scalar values that have been assigned to a character typically also have a name, such as LATIN SMALL LETTER A
and FRONT-FACING BABY CHICK
in the examples above.
全ての21ビットのユニコードスカラー値がひとつの文字に割り当てられる訳ではないことに注意してください ― いくつかのスカラーは将来の割り当てのためにまたはUTF-16符号化で使うために取っておかれます。ある文字に割り当てられるスカラー値は、たいてい名前を持っています、例えば以前の例でのLATIN SMALL LETTER A
とFRONT-FACING BABY CHICK
など。
Extended Grapheme Clusters¶ 拡張書記素クラスタ¶
Every instance of Swift’s Character
type represents a single extended grapheme cluster. An extended grapheme cluster is a sequence of one or more Unicode scalars that (when combined) produce a single human-readable character.
スウィフトのCharacter
型の全てのインスタンスは、単一の拡張書記素クラスタ(房、群)を表わします。ある拡張書記素クラスタは1つ以上のユニコード・スカラーの連なりです、それは(組み合わされて)人間の読み取り可能な1つの文字を作り出します。
Here’s an example. The letter é
can be represented as the single Unicode scalar é
(LATIN SMALL LETTER E WITH ACUTE
, or U+00E9
). However, the same letter can also be represented as a pair of scalars—a standard letter e
(LATIN SMALL LETTER E
, or U+0065
), followed by the COMBINING ACUTE ACCENT
scalar (U+0301
). The COMBINING ACUTE ACCENT
scalar is graphically applied to the scalar that precedes it, turning an e
into an é
when it’s rendered by a Unicode-aware text-rendering system.
ここにひとつの例があります。文字é
は、単一のユニコード・スカラーé
(LATIN SMALL LETTER E WITH ACUTE
、またはU+00E9
)として表わされることが出来ます。しかし、同じ文字はまた、一対のスカラー ― 通常の文字e
(LATIN SMALL LETTER E
、またはU+0065
)、それに続けてCOMBINING ACUTE ACCENT
スカラー(U+0301
)として表わされることも出来ます。COMBINING ACUTE ACCENT
スカラーは、それの前のスカラーに追加筆記され、e
を、それがユニコードに通じたテキスト描画システムによって表わされる時に、é
にします。
In both cases, the letter é
is represented as a single Swift Character
value that represents an extended grapheme cluster. In the first case, the cluster contains a single scalar; in the second case, it’s a cluster of two scalars:
両方の場合で、文字é
は、単一のスウィフトのCharacter
値として表わされます、それはひとつの拡張書記素クラスタを表わします。最初の場合では、クラスタは、ただ1つだけのスカラーを含みます;2番目の場合には、それは、2つのスカラーのクラスタ(1つの房、群れ)です:
- let eAcute: Character = "\u{E9}" // é
- let combinedEAcute: Character = "\u{65}\u{301}" // e followed by ́// e に続けて ́
- // eAcute is é, combinedEAcute is é(eAcuteはéです、combinedEAcuteはéです)
Extended grapheme clusters are a flexible way to represent many complex script characters as a single Character
value. For example, Hangul syllables from the Korean alphabet can be represented as either a precomposed or decomposed sequence. Both of these representations qualify as a single Character
value in Swift:
拡張書記素クラスタは、多くの複雑な書き方の文字を単一のCharacter
値として表わす適応性に富む方法です。例えば、コリアン・アルファベットのハングル音節は、最初から組み立てられるか、または分解されて並べたもののどちらでも表わすことが出来ます。これらの表し方の双方とも、スウィフトでは単一のCharacter
値としての基準を満たします:
- let precomposed: Character = "\u{D55C}" // 한
- let decomposed: Character = "\u{1112}\u{1161}\u{11AB}" // ᄒ, ᅡ, ᆫ
- // precomposed is 한, decomposed is 한(合成済は「한」, 分解したものは「한」です)
Extended grapheme clusters enable scalars for enclosing marks (such as COMBINING ENCLOSING CIRCLE
, or U+20DD
) to enclose other Unicode scalars as part of a single Character
value:
拡張書記素クラスタは、囲み記号としてのスカラー(COMBINING ENCLOSING CIRCLE
、またはU+20DD
)を可能にして、単一のCharacter
値の一部として他のユニコード・スカラーを囲み入れます。
- let enclosedEAcute: Character = "\u{E9}\u{20DD}"
- // enclosedEAcute is é⃝(enclosedEAcute は é⃝ です)
Unicode scalars for regional indicator symbols can be combined in pairs to make a single Character
value, such as this combination of REGIONAL INDICATOR SYMBOL LETTER U
(U+1F1FA
) and REGIONAL INDICATOR SYMBOL LETTER S
(U+1F1F8
):
地域標識記号のためのユニコード・スカラーは、単一のCharacter
値を作るために2つ一組で組み合わされることが出来ます、例えばREGIONAL INDICATOR SYMBOL LETTER U
(U+1F1FA
)とREGIONAL INDICATOR SYMBOL LETTER S
(U+1F1F8
)の組み合わせのように:
- let regionalIndicatorForUS: Character = "\u{1F1FA}\u{1F1F8}"
- // regionalIndicatorForUS is 🇺🇸(regionalIndicatorForUS は 🇺🇸 です)
Counting Characters¶ 文字を数える¶
To retrieve a count of the Character
values in a string, use the count
property of the string:
ある文字列の中のCharacter
値の総数を取り出すために、その文字列のcount
プロパティを使ってください:
- let unusualMenagerie = "Koala 🐨, Snail 🐌, Penguin 🐧, Dromedary 🐪"
- print("unusualMenagerie has \(unusualMenagerie.count) characters")
- // Prints "unusualMenagerie has 40 characters"(「unusualMenagerieは、40の文字を持ちます」を出力します)
Note that Swift’s use of extended grapheme clusters for Character
values means that string concatenation and modification may not always affect a string’s character count.
スウィフトのCharacter
値に対する拡張書記素クラスタの使用は、文字列の連結と修正が常に文字列の文字数に影響を与えないことを意味します。
For example, if you initialize a new string with the four-character word cafe
, and then append a COMBINING ACUTE ACCENT
(U+0301
) to the end of the string, the resulting string will still have a character count of 4
, with a fourth character of é
, not e
:
例えば、あなたがある新しい文字列を4文字の単語cafe
で初期化して、それからCOMBINING ACUTE ACCENT
(U+0301
)をその文字列の終わりに加えたならば、結果の文字列は依然として文字数4
で、e
ではなく、é
の4番目の文字を持ちます:>
- var word = "cafe"
- print("the number of characters in \(word) is \(word.count)")
- // Prints "the number of characters in cafe is 4"(「cafeの文字数は4です」を出力します)
- word += "\u{301}" // COMBINING ACUTE ACCENT, U+0301(アキュート・アクセント、U+0301を追加する)
- print("the number of characters in \(word) is \(word.count)")
- // Prints "the number of characters in café is 4"(「caféの文字数は4です」を出力します)
Note 注意
Extended grapheme clusters can be composed of multiple Unicode scalars. This means that different characters—and different representations of the same character—can require different amounts of memory to store. Because of this, characters in Swift don’t each take up the same amount of memory within a string’s representation. As a result, the number of characters in a string can’t be calculated without iterating through the string to determine its extended grapheme cluster boundaries. If you are working with particularly long string values, be aware that the count
property must iterate over the Unicode scalars in the entire string in order to determine the characters for that string.
拡張書記素クラスタは、多数のユニコード・スカラーから構成される可能性があります。これは異なる文字—さらに同じ文字の異なる表現—が、格納するために異なる量のメモリを必要とすることがあるのを意味します。これのため、スウィフトの中の文字は、それぞれが同じ量のメモリを文字列の表現において取るわけではありません。その結果、ある文字列の中の文字の数は、それの拡張書記素クラスタ境界を解決するためにその文字列の最初から終わりまで繰り返していくことなしに計算されることができません。あなたが特に長い文字列値を扱う場合は、count
プロパティは、その文字列の文字を決定する目的で文字列全体のユニコード・スカラーのすべてに繰り返しを行わなければならないことを知っていてください。
The count of the characters returned by the count
property isn’t always the same as the length
property of an NSString
that contains the same characters. The length of an NSString
is based on the number of 16-bit code units within the string’s UTF-16 representation and not the number of Unicode extended grapheme clusters within the string.
count
プロパティによって返される文字の総数は、同じ文字を含むNSString
のlength
プロパティと常に同じではありません。あるNSString
の長さは、その文字列のUTF-16表現内の16ビットコード単位の数に基づきます、その文字列内のユニコード拡張書記素クラスタの数ではありません。
Accessing and Modifying a String¶ 文字列へのアクセスと修正¶
You access and modify a string through its methods and properties, or by using subscript syntax. あなたは文字列へのアクセスと修正を、それのメソッドとプロパティを通して、または添え字構文を使うことによって行います。
String Indices¶ 文字列インデックス¶
Each String
value has an associated index type, String.Index
, which corresponds to the position of each Character
in the string.
それぞれのString
値は、結び付けられたインデックス型、String.Index
を持ちます、それは、各Character
のその文字列中での位置に対応しています。
As mentioned above, different characters can require different amounts of memory to store, so in order to determine which Character
is at a particular position, you must iterate over each Unicode scalar from the start or end of that String
. For this reason, Swift strings can’t be indexed by integer values.
上で述べたように、異なる文字は格納するのに異なるメモリ量を必要とすることがあり得ます、それでCharacter
がある特定の位置にあることを確定するために、あなたはそのString
の始まりまたは終わりからユニコードスカラーそれぞれにわたってずっと繰り返さなければなりません。この理由のために、スウィフトの文字列は整数値でインデックス付けされることができません。
Use the startIndex
property to access the position of the first Character
of a String
. The endIndex
property is the position after the last character in a String
. As a result, the endIndex
property isn’t a valid argument to a string’s subscript. If a String
is empty, startIndex
and endIndex
are equal.
あるString
の最初のCharacter
の位置にアクセスするためにstartIndex
プロパティを使ってください。endIndex
プロパティは、あるString
の最後の文字の後の位置を返します。結果として、endIndex
プロパティは、文字列の添え字として有効な引数ではありません。あるString
が空ならば、startIndex
とendIndex
は同じです。
You access the indices before and after a given index using the index(before:)
and index(after:)
methods of String
. To access an index farther away from the given index, you can use the index(_:offsetBy:)
method instead of calling one of these methods multiple times.
あなたは、ある与えられたインデックスの前後のインデックスにString
のindex(before:)
とindex(after:)
メソッドを使ってアクセスします。与えられたインデックスからもっと遠く離れたインデックスにアクセスするには、あなたはこれらのメソッドのうちの1つを複数回呼び出すことの代わりにindex(_:offsetBy:)
メソッドを使うことができます。
You can use subscript syntax to access the Character
at a particular String
index.
あなたは、添え字構文を使うことで特定のString
インデックス位置でのCharacter
へアクセスできます。
- let greeting = "Guten Tag!"
- greeting[greeting.startIndex]
- // G
- greeting[greeting.index(before: greeting.endIndex)]
- // !
- greeting[greeting.index(after: greeting.startIndex)]
- // u
- let index = greeting.index(greeting.startIndex, offsetBy: 7)
- greeting[index]
- // a
Attempting to access an index outside of a string’s range or a Character
at an index outside of a string’s range will trigger a runtime error.
ある文字列の範囲外のインデックスにまたはある文字列の範囲外のインデックスでCharacter
にアクセスを試みることは、実行時エラーの引き金となります。
- greeting[greeting.endIndex] // Error
- greeting.index(after: greeting.endIndex) // Error
Use the indices
property to access all of the indices of individual characters in a string.
indices
プロパティを使って、ある文字列中の個々の文字のインデックスの全てにアクセスしてください。
- for index in greeting.indices {
- print("\(greeting[index]) ", terminator: "")
- }
- // Prints "G u t e n T a g ! "
Note 注意
You can use the startIndex
and endIndex
properties and the index(before:)
, index(after:)
, and index(_:offsetBy:)
methods on any type that conforms to the Collection
protocol. This includes String
, as shown here, as well as collection types such as Array
, Dictionary
, and Set
.
あなたは、startIndex
とendIndex
プロパティそしてindex(before:)
、index(after:)
、およびindex(_:offsetBy:)メ
ソッドをCollection
プロトコルに準拠するあらゆる型で使うことができます。これは、ここで示すようにString
を、それだけでなくArray
、Dictionary
、そしてSet
といったコレクション型も含みます。
Inserting and Removing¶ 差し込みと削除¶
To insert a single character into a string at a specified index, use the insert(_:at:)
method, and to insert the contents of another string at a specified index, use the insert(contentsOf:at:)
method.
ある単一の文字をある文字列中へ特定のインデックスで差し込むには、insert(_:at:)
メソッドを使ってください、そして別の文字列の内容を特定のインデックスで差し込むには、insert(contentsOf:at:)
メソッドを使ってください。
- var welcome = "hello"
- welcome.insert("!", at: welcome.endIndex)
- // welcome now equals "hello!"(welcomeは、現在「こんにちは!」に等しい)
- welcome.insert(contentsOf: " there", at: welcome.index(before: welcome.endIndex))
- // welcome now equals "hello there!"(welcomeは、現在「やあ!、こんにちは」に等しい)
To remove a single character from a string at a specified index, use the remove(at:)
method, and to remove a substring at a specified range, use the removeSubrange(_:)
method:
ある単一の文字をある文字列から特定のインデックスで削除するには、remove(at:)
メソッドを使ってください、そして部分文字列を特定の範囲で削除するには、removeSubrange(_:)
メソッドを使ってください。
- welcome.remove(at: welcome.index(before: welcome.endIndex))
- // welcome now equals "hello there"(welcomeは、現在「やあ、こんにちは」に等しい)
- let range = welcome.index(welcome.endIndex, offsetBy: -6)..<welcome.endIndex
- welcome.removeSubrange(range)
- // welcome now equals "hello"(welcomeは、現在「こんにちは」に等しい)
Note 注意
You can use the insert(_:at:)
, insert(contentsOf:at:)
, remove(at:)
, and removeSubrange(_:)
methods on any type that conforms to the RangeReplaceableCollection
protocol. This includes String
, as shown here, as well as collection types such as Array
, Dictionary
, and Set
.
あなたは、insert(_:at:)
、insert(contentsOf:at:)
、remove(at:)
、そしてremoveSubrange(_:)
メソッドをRangeReplaceableCollection
プロトコルに準拠するあらゆる型で使うことができます。これは、ここで示すようにString
を、それだけでなくArray
、Dictionary
、そしてSet
といったコレクション型も含みます。
Substrings¶ 下位文字列¶
When you get a substring from a string—for example, using a subscript or a method like prefix(_:)
—the result is an instance of Substring
, not another string. Substrings in Swift have most of the same methods as strings, which means you can work with substrings the same way you work with strings. However, unlike strings, you use substrings for only a short amount of time while performing actions on a string. When you’re ready to store the result for a longer time, you convert the substring to an instance of String
. For example:
あなたがある文字列から下位文字列を得る場合 — 例えば、添え字またはprefix(_:)
のようなメソッドを使って — その結果はSubstring
のインスタンスとなります、別の文字列ではなく。スウィフトでの下位文字列は、文字列とほとんど同じメソッドを持ちます、それはあなたが下位文字列を、文字列を扱うのと同じ方法で扱えることを意味します。しかしながら、文字列と違い、ある文字列に関して様々な行為を行う間の短いある程度の時間だけのために、あなたは下位文字列を使います。あなたが結果を長期間にわたって格納しようとする場合、あなたは下位文字列をString
のインスタンスへと変換します。例えば:
- let greeting = "Hello, world!"
- let index = greeting.firstIndex(of: ",") ?? greeting.endIndex
- let beginning = greeting[..<index]
- // beginning is "Hello"(beginningは "Hello" です)
- // Convert the result to a String for long-term storage.(結果を長期保管のためのStringへ変換する。)
- let newString = String(beginning)
Like strings, each substring has a region of memory where the characters that make up the substring are stored. The difference between strings and substrings is that, as a performance optimization, a substring can reuse part of the memory that’s used to store the original string, or part of the memory that’s used to store another substring. (Strings have a similar optimization, but if two strings share memory, they’re equal.) This performance optimization means you don’t have to pay the performance cost of copying memory until you modify either the string or substring. As mentioned above, substrings aren’t suitable for long-term storage—because they reuse the storage of the original string, the entire original string must be kept in memory as long as any of its substrings are being used. 文字列のように、下位文字列それぞれはメモリのある領域を持ち、そこで下位文字列を作り上げる文字が格納されます。文字列と下位文字列の違いは、性能最適化として、下位文字列はオリジナルの文字列を格納するために使われるメモリについての、または別の下位文字列を格納するために使われるメモリの一部についての再利用ができます。(文字列は同様な最適化を待ちます、しかし2つの文字列がメモリを共有するならば、それらは等しいです。)この性能最適化が意味するのは、あなたがメモリをコピーする性能経費を、あなたが文字列か下位文字列のどちらかを修正するまでは払う必要がないことです。上で言及したように、下位文字列は長期保管に適していません — それらがオリジナルの文字列のストレージを再利用することから、オリジナルの文字列全体はそれの下位文字列が使われている限りメモリに保持されなければなりません。
In the example above, greeting
is a string, which means it has a region of memory where the characters that make up the string are stored. Because beginning
is a substring of greeting
, it reuses the memory that greeting
uses. In contrast, newString
is a string—when it’s created from the substring, it has its own storage. The figure below shows these relationships:
上の例において、greeting
は文字列です、それはその文字列を作り上げる文字が格納されるところのメモリ領域をそれが持つことを意味します。beginning
がgreeting
の下位文字列であることから、それはgreeting
が使うメモリを再利用します。対照的に、newString
は文字列です — それが下位文字列から作成されるとき、それはそれ独自のストレージを持ちます。下の図は、それらの関係を示します:
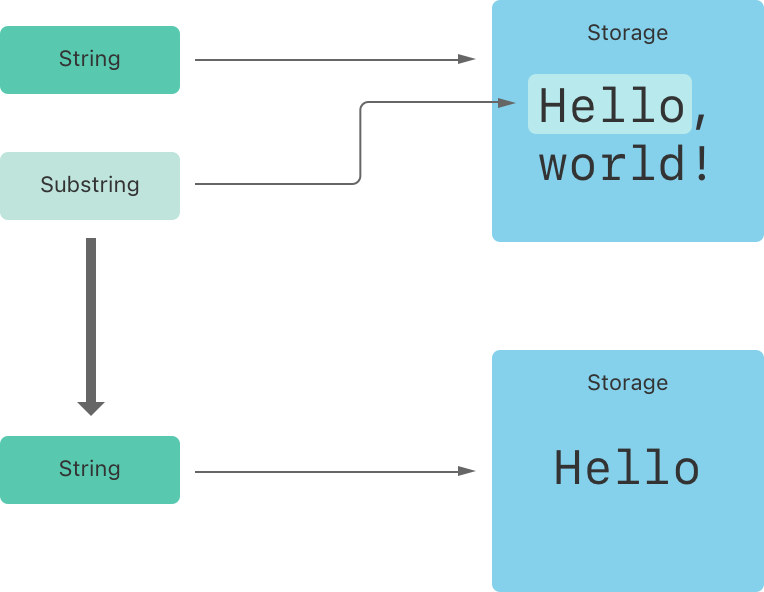
Note 注意
Both String
and Substring
conform to the StringProtocol
protocol, which means it’s often convenient for string-manipulation functions to accept a StringProtocol
value. You can call such functions with either a String
or Substring
value.
String
とSubstring
の両方ともStringProtocol
プロトコルに準拠します、それはStringProtocol
値を受け取ることは文字列操作関数それぞれにとってしばしば都合が良いのを意味します。あなたは、そのような関数をString
またはSubstring
値で呼出せます。
Comparing Strings¶ 文字列を比較する¶
Swift provides three ways to compare textual values: string and character equality, prefix equality, and suffix equality. スウィフトは、テキスト関連の値を比較する3つの方法を提供します:文字列および文字の等式、接頭辞等式、そして接尾辞等式。
String and Character Equality¶ 文字列と文字の同等性¶
String and character equality is checked with the “equal to” operator (==
) and the “not equal to” operator (!=
), as described in Comparison Operators:
文字列と文字の同等性は、「同等」演算子(==
)および「不等」演算子(!=
)で調べられます、これは比較演算子で記述されます:
- let quotation = "We're a lot alike, you and I."
- let sameQuotation = "We're a lot alike, you and I."
- if quotation == sameQuotation {
- print("These two strings are considered equal")
- }
- // Prints "These two strings are considered equal"(「これら2つの文字列は等しいと考えられる」を出力します)
Two String
values (or two Character
values) are considered equal if their extended grapheme clusters are canonically equivalent. Extended grapheme clusters are canonically equivalent if they have the same linguistic meaning and appearance, even if they’re composed from different Unicode scalars behind the scenes.
2つのString
値(または2つのCharacter
)は、それらの拡張書記素クラスタそれらが正準等価ならば、等しいとみなされます。拡張書記素クラスタが正準等価であるのは、それらが同じ言語的な意味と外観を持つ場合です、たとえそれらが舞台裏で異なるユニコードスカラーから組み立てられるとしてもです。
For example, LATIN SMALL LETTER E WITH ACUTE
(U+00E9
) is canonically equivalent to LATIN SMALL LETTER E
(U+0065
) followed by COMBINING ACUTE ACCENT
(U+0301
). Both of these extended grapheme clusters are valid ways to represent the character é
, and so they’re considered to be canonically equivalent:
例えば、LATIN SMALL LETTER E WITH ACUTE
(U+00E9
)は、LATIN SMALL LETTER E
(U+0065
)にCOMBINING ACUTE ACCENT
(U+0301
)を続けたものと正準等価です。これらの拡張書記素クラスタの両方とも、文字é
を表わす有効な方法です、そういうわけでそれらは正準等価とみなされます:
- // "Voulez-vous un café?" using LATIN SMALL LETTER E WITH ACUTE(「Voulez-vous un café?」は揚音付きラテン小文字eを使用している)
- let eAcuteQuestion = "Voulez-vous un caf\u{E9}?"
- // "Voulez-vous un café?" using LATIN SMALL LETTER E and COMBINING ACUTE ACCENT(「Voulez-vous un café?」はラテン小文字eと揚音アクセント結合を使用している)
- let combinedEAcuteQuestion = "Voulez-vous un caf\u{65}\u{301}?"
- if eAcuteQuestion == combinedEAcuteQuestion {
- print("These two strings are considered equal")
- }
- // Prints "These two strings are considered equal"(「これら2つの文字列は等しいと考えられる」を出力します)
Conversely, LATIN CAPITAL LETTER A
(U+0041
, or "A"
), as used in English, is not equivalent to CYRILLIC CAPITAL LETTER A
(U+0410
, or "А"
), as used in Russian. The characters are visually similar, but don’t have the same linguistic meaning:
反対に、英語で使われるような、LATIN CAPITAL LETTER A
(U+0041
、または"A"
)は、ロシアで使われるような、CYRILLIC CAPITAL LETTER A
(U+0410
、または"А"
)と等しくありません。これらの文字は視覚的には同じ形です、しかし同じ言語的意味を持ちません:
- let latinCapitalLetterA: Character = "\u{41}"
- let cyrillicCapitalLetterA: Character = "\u{0410}"
- if latinCapitalLetterA != cyrillicCapitalLetterA {
- print("These two characters aren't equivalent.")
- }
- // Prints "These two characters aren't equivalent."(「これら2つの文字列は等しくない。」を出力します)
Note 注意
String and character comparisons in Swift aren’t locale-sensitive. 文字列および文字の比較はスウィフトではロケールに影響されません。
Prefix and Suffix Equality¶ 接頭辞と接尾辞等式¶
To check whether a string has a particular string prefix or suffix, call the string’s hasPrefix(_:)
and hasSuffix(_:)
methods, both of which take a single argument of type String
and return a Boolean value.
ある文字列が特定の文字列接頭辞または接尾辞を持つかどうか調べるために、文字列のhasPrefix(_:)
とhasSuffix(_:)
メソッドを呼んでください、それらの両方ともただ1つのString
型の引数をとって、ブールの値を返します。
The examples below consider an array of strings representing the scene locations from the first two acts of Shakespeare’s Romeo and Juliet: 下の例は、シェークスピアのロミオとジュリエットの最初の2幕から、場面場所を表している文字列の配列を考えてみます:
- let romeoAndJuliet = [
- "Act 1 Scene 1: Verona, A public place",
- "Act 1 Scene 2: Capulet's mansion",
- "Act 1 Scene 3: A room in Capulet's mansion",
- "Act 1 Scene 4: A street outside Capulet's mansion",
- "Act 1 Scene 5: The Great Hall in Capulet's mansion",
- "Act 2 Scene 1: Outside Capulet's mansion",
- "Act 2 Scene 2: Capulet's orchard",
- "Act 2 Scene 3: Outside Friar Lawrence's cell",
- "Act 2 Scene 4: A street in Verona",
- "Act 2 Scene 5: Capulet's mansion",
- "Act 2 Scene 6: Friar Lawrence's cell"
- ]
You can use the hasPrefix(_:)
method with the romeoAndJuliet
array to count the number of scenes in Act 1 of the play:
あなたは、劇の「一幕(Act 1)」の場面の数を数えるためにhasPrefix(_:)
メソッドをromeoAndJuliet
配列で使用することができます:
- var act1SceneCount = 0
- for scene in romeoAndJuliet {
- if scene.hasPrefix("Act 1 ") {
- act1SceneCount += 1
- }
- }
- print("There are \(act1SceneCount) scenes in Act 1")
- // Prints "There are 5 scenes in Act 1"(「5つの場面が一幕にあります」を出力します)
Similarly, use the hasSuffix(_:)
method to count the number of scenes that take place in or around Capulet’s mansion and Friar Lawrence’s cell:
同じように、hasSuffix(_:)
メソッドを、キャビュレットの邸宅と修道士ローレンスの独居房の中または周囲で起こる場面の数を数えるために使用してください:
- var mansionCount = 0
- var cellCount = 0
- for scene in romeoAndJuliet {
- if scene.hasSuffix("Capulet's mansion") {
- mansionCount += 1
- } else if scene.hasSuffix("Friar Lawrence's cell") {
- cellCount += 1
- }
- }
- print("\(mansionCount) mansion scenes; \(cellCount) cell scenes")
- // Prints "6 mansion scenes; 2 cell scenes"(「6つの邸宅場面;2つの僧房場面」を出力します)
Note 注意
The hasPrefix(_:)
and hasSuffix(_:)
methods perform a character-by-character canonical equivalence comparison between the extended grapheme clusters in each string, as described in String and Character Equality.
hasPrefix(_:)
とhasSuffix(_:)
は、各文字列の拡張書記素クラスタ間で文字ごとの正準等価比較を実行します、そのことは文字列と文字の同等性で記述されます。
Unicode Representations of Strings¶ 文字列のユニコード表現¶
When a Unicode string is written to a text file or some other storage, the Unicode scalars in that string are encoded in one of several Unicode-defined encoding forms. Each form encodes the string in small chunks known as code units. These include the UTF-8 encoding form (which encodes a string as 8-bit code units), the UTF-16 encoding form (which encodes a string as 16-bit code units), and the UTF-32 encoding form (which encodes a string as 32-bit code units). あるUnicode文字列がテキスト・ファイルまたは何か他の記憶装置に書かれるとき、その文字列内のユニコード・スカラーはいくつかのUnicode定義の符号化方式のうちの1つで符号化されます。各方式は、文字列を、符号単位として知られている小さいかたまりで符号化します。これらは、UTF-8符号化方式(それは、8ビット符号単位として文字列を符号化します)、UTF-16符号化方式(それは、16ビット符号単位として文字列を符号化します)、そしてUTF-32符号化方式(それは、32ビット符号単位として文字列を符号化します)を含みます。
Swift provides several different ways to access Unicode representations of strings. You can iterate over the string with a for
-in
statement, to access its individual Character
values as Unicode extended grapheme clusters. This process is described in Working with Characters.
スウィフトは、文字列のUnicode表現にアクセスするためにいくつかの異なる方法を提供します。あなたは文字列の全体にわたってfor
-in
文を使って繰り返すことができます、それでユニコードの拡張書記素クラスタとしてのそれの個々のCharacter
値にアクセスします。このやり方は文字を扱うで記述されます。
Alternatively, access a String
value in one of three other Unicode-compliant representations:
あるいは代わりに、3つの他のUnicode対応の表現の1つでString
値にアクセスしてください:
- A collection of UTF-8 code units (accessed with the string’s
utf8
property) UTF-8符号単位の集まり(文字列のutf8
プロパティでアクセスされます) - A collection of UTF-16 code units (accessed with the string’s
utf16
property) UTF-16符号単位の集まり(文字列のutf16
プロパティでアクセスされます) - A collection of 21-bit Unicode scalar values, equivalent to the string’s UTF-32 encoding form (accessed with the string’s
unicodeScalars
property) 21ビットのUnicodeスカラー値の集まり、その文字列のUTF-32符号化方式と等しい(文字列のunicodeScalars
プロパティでアクセスされます)
Each example below shows a different representation of the following string, which is made up of the characters D
, o
, g
, ‼
(DOUBLE EXCLAMATION MARK
, or Unicode scalar U+203C
), and the 🐶 character (DOG FACE
, or Unicode scalar U+1F436
):
以下の各例は、次の文字列の異なる表現を示します、それは文字D
、o
、g
、‼
(DOUBLE EXCLAMATION MARK
、またはユニコードスカラーU+203C
)、そして🐶文字(DOG FACE
またはユニコードスカラーU+1F436
)から成ります:
- let dogString = "Dog‼🐶"
UTF-8 Representation¶ UTF-8表現¶
You can access a UTF-8 representation of a String
by iterating over its utf8
property. This property is of type String.UTF8View
, which is a collection of unsigned 8-bit (UInt8
) values, one for each byte in the string’s UTF-8 representation:
あなたは、あるString
のUTF-8叙述に、それのutf8
プロパティのすべてに渡って繰り返していくことによってアクセスすることができます。このプロパティは型String.UTF8View
です、そしてそれは、符号なし8ビット(UInt8
)の値の集まりで、それぞれがその文字列のUTF-8叙述における各バイトです:
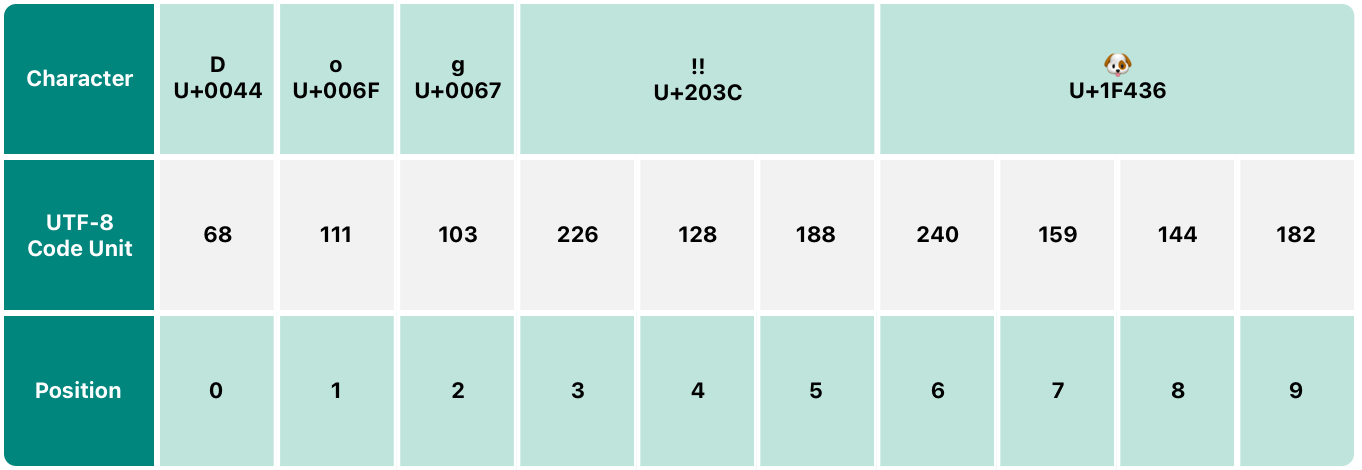
- for codeUnit in dogString.utf8 {
- print("\(codeUnit) ", terminator: "")
- }
- print("")
- // Prints "68 111 103 226 128 188 240 159 144 182 "
In the example above, the first three decimal codeUnit
values (68
, 111
, 103
) represent the characters D
, o
, and g
, whose UTF-8 representation is the same as their ASCII representation. The next three decimal codeUnit
values (226
, 128
, 188
) are a three-byte UTF-8 representation of the DOUBLE EXCLAMATION MARK
character. The last four codeUnit
values (240
, 159
, 144
, 182
) are a four-byte UTF-8 representation of the DOG FACE
character.
上の例において、最初の3つの10進のcodeUnit
値(68
、111
、103
)は、文字D
、o
、そしてg
を表わします、これらのUTF-8叙述はそれらのASCII叙述と同じものです。次の3つの10進のcodeUnit
値(226
、128
、188
)は、DOUBLE EXCLAMATION MARK
文字の3バイトUTF-8叙述です。最後の4つのcodeUnit
値(240
、159
、144
、182
)は、DOG FACE
文字の4バイトUTF-8叙述です。
UTF-16 Representation¶ UTF-16表現¶
You can access a UTF-16 representation of a String
by iterating over its utf16
property. This property is of type String.UTF16View
, which is a collection of unsigned 16-bit (UInt16
) values, one for each 16-bit code unit in the string’s UTF-16 representation:
あなたは、あるString
のUTF-16叙述に、それのutf16
プロパティのすべてに渡って繰り返していくことによってアクセスすることができます。このプロパティは型String.UTF16View
です、そしてそれは、符号なし16ビット(UInt16
)の値の集まりで、そのひとつがその文字列のUTF-16叙述における各16ビット符号単位です:
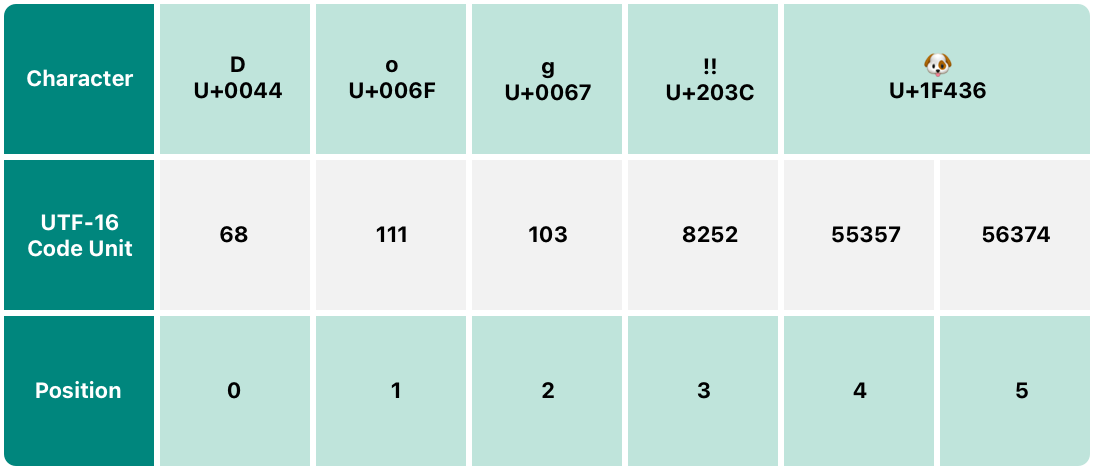
- for codeUnit in dogString.utf16 {
- print("\(codeUnit) ", terminator: "")
- }
- print("")
- // Prints "68 111 103 8252 55357 56374 "
Again, the first three codeUnit
values (68
, 111
, 103
) represent the characters D
, o
, and g
, whose UTF-16 code units have the same values as in the string’s UTF-8 representation (because these Unicode scalars represent ASCII characters).
再び、最初の3つのcodeUnit
値(68
、111
、103
)は文字D
、o
、そしてg
を表します、これらのUTF-16符号単位はこの文字列のUTF-8叙述の場合と同じ値を持ちます(なぜなら、これらのユニコード・スカラーがASCII文字を表わすからです)。
The fourth codeUnit
value (8252
) is a decimal equivalent of the hexadecimal value 203C
, which represents the Unicode scalar U+203C
for the DOUBLE EXCLAMATION MARK
character. This character can be represented as a single code unit in UTF-16.
4番目のcodeUnit
値(8252
)は、16進数の値203C
です、それは、DOUBLE EXCLAMATION MARK
文字に対するユニコード・スカラーU+203C
を表わします。この文字は、UTF-16において単一の符号単位で表わされることが出来ます。
The fifth and sixth codeUnit
values (55357
and 56374
) are a UTF-16 surrogate pair representation of the DOG FACE
character. These values are a high-surrogate value of U+D83D
(decimal value 55357
) and a low-surrogate value of U+DC36
(decimal value 56374
).
5番目と6番目のcodeUnit
値(55357
と56374
)は、DOG FACE
文字のUTF-16代用対叙述です。これらの値は、前半代用値のU+D83D
(10進の値55357
)と後半代用値のU+DC36
(10進の値56374
)です。
Unicode Scalar Representation¶ ユニコード・スカラー表現¶
You can access a Unicode scalar representation of a String
value by iterating over its unicodeScalars
property. This property is of type UnicodeScalarView
, which is a collection of values of type UnicodeScalar
.
あなたは、あるString
のUnicodeスカラー叙述に、それのunicodeScalars
プロパティのすべてに渡って繰り返していくことによってアクセスすることができます。このプロパティは型UnicodeScalarView
です、そしてそれは、UnicodeScalar
型の値の集まりです。
Each UnicodeScalar
has a value
property that returns the scalar’s 21-bit value, represented within a UInt32
value:
各UnicodeScalar
はひとつのvalue
プロパティを持ちます、それはそのスカラーの21ビットの値を返します、そしてそれはUInt32
値の範囲内で表されます:
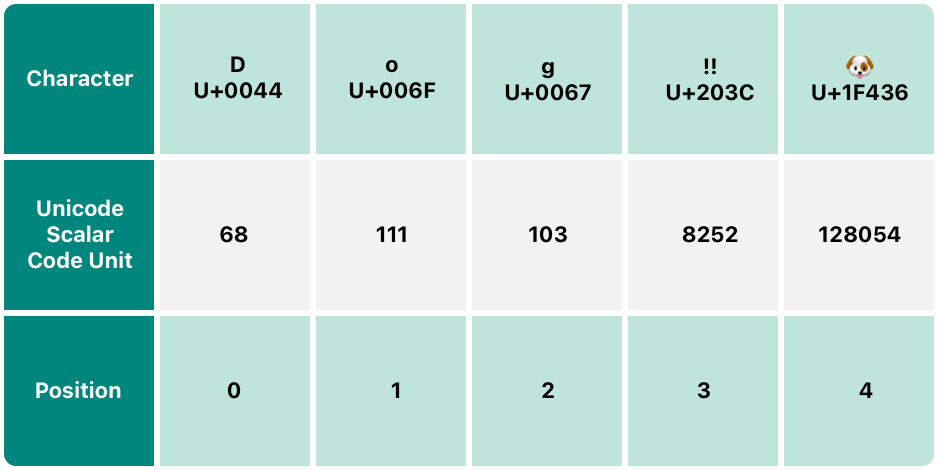
- for scalar in dogString.unicodeScalars {
- print("\(scalar.value) ", terminator: "")
- }
- print("")
- // Prints "68 111 103 8252 128054 "
The value
properties for the first three UnicodeScalar
values (68
, 111
, 103
) once again represent the characters D
, o
, and g
.
最初の3つのUnicodeScalar
値(68
、111
、103
)のためのvalue
プロパティは、またふたたび文字D
、o
、そしてg
を表します。
The fourth codeUnit
value (8252
) is again a decimal equivalent of the hexadecimal value 203C
, which represents the Unicode scalar U+203C
for the DOUBLE EXCLAMATION MARK
character.
4番目のcodeUnit
値(8252
)は、ふたたび16進数値203C
の10進の等価物です、それは、DOUBLE EXCLAMATION MARK
文字に対するユニコード・スカラーU+203C
を表わします。
The value
property of the fifth and final UnicodeScalar
, 128054
, is a decimal equivalent of the hexadecimal value 1F436
, which represents the Unicode scalar U+1F436
for the DOG FACE
character.
5番目で最後のUnicodeScalar
のvalue
プロパティ、128054
は、16進の値1F436
の10進の等価物です、そしてそれは、DOG FACE
文字のためのユニコード・スカラーU+1F436
を表わします。
As an alternative to querying their value
properties, each UnicodeScalar
value can also be used to construct a new String
value, such as with string interpolation:
それらのvalue
プロパティについて尋ねることに代わるものとして、各UnicodeScalar
値は、また、新しいString
値を造るために使われることもできます、例えば文字列補間を使って:
- for scalar in dogString.unicodeScalars {
- print("\(scalar) ")
- }
- // D
- // o
- // g
- // ‼
- // 🐶