Subscripts¶ 添え字¶
Classes, structures, and enumerations can define subscripts, which are shortcuts for accessing the member elements of a collection, list, or sequence. You use subscripts to set and retrieve values by index without needing separate methods for setting and retrieval. For example, you access elements in an Array
instance as someArray[index]
and elements in a Dictionary
instance as someDictionary[key]
.
クラス、構造体、および列挙は、添え字を定義することができます、それは、コレクション、リスト、またはシーケンスのメンバー要素にアクセスするための近道です。あなたは添え字を使うことで、独立したメソッドを設定や検索のために必要とすることなしに、インデックスによって値を設定したり取得したりします。例えば、あなたはあるArray
インスタンスの中の要素にsomeArray[index]
として、そしてあるDictionary
インスタンスの中の要素にsomeDictionary[key]
のようにアクセスします。
You can define multiple subscripts for a single type, and the appropriate subscript overload to use is selected based on the type of index value you pass to the subscript. Subscripts aren’t limited to a single dimension, and you can define subscripts with multiple input parameters to suit your custom type’s needs. あなたは1つの型のために複数の添え字を定義することができます、そしてあなたが添え字に渡すインデックス値の型に基づいて、使うのに適切な添え字のオーバーロードが選択されます。添え字は1つの次元に制限されません、なのであなたは複数の入力パラメータをもつ添え字を定義して、あなたのあつらえの型の必要を満たすことが出来ます。
Subscript Syntax¶ 添え字の構文¶
Subscripts enable you to query instances of a type by writing one or more values in square brackets after the instance name. Their syntax is similar to both instance method syntax and computed property syntax. You write subscript definitions with the subscript
keyword, and specify one or more input parameters and a return type, in the same way as instance methods. Unlike instance methods, subscripts can be read-write or read-only. This behavior is communicated by a getter and setter in the same way as for computed properties:
添え字は、あなたにそのインスタンス名の後で角括弧の中に1つ以上の値を書くことによって、ある型のインスタンスに問い合わせることを可能にします。それらの構文は、インスタンスメソッド構文と計算プロパティ構文に似ています。あなたは、添え字定義をsubscript
キーワードを使って書きます、そしてインスタンスメソッドと同じ方法で、1つ以上の入力パラメータと戻り型を指定します。インスタンスメソッドと違って、添え字は読み書き用であるか読み出し専用であることができます。この挙動は、計算プロパティに関してと同様に、ゲッターとセッターによって伝えられます:
- subscript(index: Int) -> Int {
- get {
- // Return an appropriate subscript value here.(適切な添え字値をここで返す。)
- }
- set(newValue) {
- // Perform a suitable setting action here.(ふさわしい設定動作をここで行う。)
- }
- }
The type of newValue
is the same as the return value of the subscript. As with computed properties, you can choose not to specify the setter’s (newValue)
parameter. A default parameter called newValue
is provided to your setter if you don’t provide one yourself.
newValue
の型は、添え字の戻り値と同じものです。計算プロパティと同様に、あなたはセッターの(newValue)
パラメータを指定しないほうを選ぶことができます。あなたが独自のものを提供しないならば、newValue
と呼ばれる省略時のパラメータがあなたのセッターに提供されます。
As with read-only computed properties, you can simplify the declaration of a read-only subscript by removing the get
keyword and its braces:
読み出し専用の計算プロパティでのように、あなたは読み出し専用の添え字の宣言を単純化することが、get
キーワードとそれの波括弧を取り除くことによって行えます:
- subscript(index: Int) -> Int {
- // Return an appropriate subscript value here.(適切な添え字値をここで返す。)
- }
Here’s an example of a read-only subscript implementation, which defines a TimesTable
structure to represent an n-times-table of integers:
ここに読み出し専用の添え字の実施の例があります、それは、整数の九九のn段を表すTimesTable
構造体を定義します:
- struct TimesTable {
- let multiplier: Int
- subscript(index: Int) -> Int {
- return multiplier * index
- }
- }
- let threeTimesTable = TimesTable(multiplier: 3)
- print("six times three is \(threeTimesTable[6])")
- // Prints "six times three is 18"(「6かける3は18です」を出力します)
In this example, a new instance of TimesTable
is created to represent the three-times-table. This is indicated by passing a value of 3
to the structure’s initializer
as the value to use for the instance’s multiplier
parameter.
この例では、TimesTable
の新しいインスタンスは、九九の3の段を表すためにつくられます。これは、値3
をこの構造体のinitializer
にインスタンスのmultiplier
パラメータのために使う値として渡すことによって示されます。
You can query the threeTimesTable
instance by calling its subscript, as shown in the call to threeTimesTable[6]
. This requests the sixth entry in the three-times-table, which returns a value of 18
, or 3
times 6
.
あなたは、threeTimesTable
インスタンスにその添え字を呼ぶことによって問い合わせることがthreeTimesTable[6]
への呼び出しで示されるように可能です。これは九九の3の段において6番目の部分を要請します、それは値18
、つまり3
掛ける6
を返します。
Note 注意
An n-times-table is based on a fixed mathematical rule. It isn’t appropriate to set threeTimesTable[someIndex]
to a new value, and so the subscript for TimesTable
is defined as a read-only subscript.
九九のnの段は、決められた数学的な規則に基づきます。threeTimesTable[someIndex]
を新しい値に設定することは適切ではありません、なのでTimesTable
のための添え字は、読み出し専用の添え字として定義されます。
Subscript Usage¶ 添え字の使用法¶
The exact meaning of “subscript” depends on the context in which it’s used. Subscripts are typically used as a shortcut for accessing the member elements in a collection, list, or sequence. You are free to implement subscripts in the most appropriate way for your particular class or structure’s functionality. 「添え字」の正確な意味は、それが使われる前後関係に依存します。添え字は、一般的に、コレクション、リスト、またはシーケンスの中のメンバー要素にアクセスするための近道として使われます。あなたは、あなたの特定のクラスまたは構造体の機能性に最も適切な方法で、添え字を実装して結構です。
For example, Swift’s Dictionary
type implements a subscript to set and retrieve the values stored in a Dictionary
instance. You can set a value in a dictionary by providing a key of the dictionary’s key type within subscript brackets, and assigning a value of the dictionary’s value type to the subscript:
例えば、スウィフトのDictionary
型は、Dictionary
インスタンスに格納される値を設定したり取り出すために添え字を実装します。あなたは、添え字角括弧内にその辞書のキー型のキーを提供し、そしてその添え字に辞書の値型の値を代入することによって、値を辞書に設定することができます:
- var numberOfLegs = ["spider": 8, "ant": 6, "cat": 4]
- numberOfLegs["bird"] = 2
The example above defines a variable called numberOfLegs
and initializes it with a dictionary literal containing three key-value pairs. The type of the numberOfLegs
dictionary is inferred to be [String: Int]
. After creating the dictionary, this example uses subscript assignment to add a String
key of "bird"
and an Int
value of 2
to the dictionary.
上の例は、numberOfLegs
と呼ばれる変数を定義して、3つの「キーと値」の対を含んでいる辞書リテラルでそれを初期化します。numberOfLegs
辞書の型は、[String: Int]
であると推測されます。辞書を作成した後に、この例は、辞書にString
キーの"bird"
とInt
値の2
を加えるために添え字代入を使います。
For more information about Dictionary
subscripting, see Accessing and Modifying a Dictionary.
Dictionary
で添え字を使うことの詳細については、辞書へのアクセスと修正を見てください。
Note 注意
Swift’s Dictionary
type implements its key-value subscripting as a subscript that takes and returns an optional type. For the numberOfLegs
dictionary above, the key-value subscript takes and returns a value of type Int?
, or “optional int”. The Dictionary
type uses an optional subscript type to model the fact that not every key will have a value, and to give a way to delete a value for a key by assigning a nil
value for that key.
スウィフトのDictionary
型は、それの「キーと値」に関する添え字を、オプショナル型を受け取り返す添え字として実装します。上のnumberOfLegs
辞書のために、その「キーと値」添え字は型Int?
、つまり「オプショナルのint」の値を受け取りそして返します。Dictionary
型は、すべてのキーに値があるというわけでないという事実をモデル化するために、そしてあるキーに対する値を削除する方法をそのキーに対してnil
の値を代入することで提供するために、オプショナルの添え字型を使います。
Subscript Options¶ 添え字オプション¶
Subscripts can take any number of input parameters, and these input parameters can be of any type. Subscripts can also return a value of any type. 添え字は、任意の数の入力パラメータをとることができます、そしてこれらの入力パラメータはどんな型でもかまいません。添え字はまた、任意の型の値を返すことができます。
Like functions, subscripts can take a varying number of parameters and provide default values for their parameters, as discussed in Variadic Parameters and Default Parameter Values. However, unlike functions, subscripts can’t use in-out parameters. 関数のように、添え字は可変数のパラメータを取りそしてそれらのパラメータに対して省略時の値を提供できます、可変長パラメータおよび省略時のパラメータ値で議論されるように。しかしながら、関数とは違い、添え字はin-outパラメータを使用できません。
A class or structure can provide as many subscript implementations as it needs, and the appropriate subscript to be used will be inferred based on the types of the value or values that are contained within the subscript brackets at the point that the subscript is used. This definition of multiple subscripts is known as subscript overloading. クラスや構造体はそれが必要とする多くの添え字実装を提供することが可能です、そして使用されるのに適切な添え字が、添え字が使われる時点でその添え字角括弧内に含まれる値または複数の値の型に基づいて、推論されます。この複数の添え字の定義は、添え字オーバーロードとして知られています。
While it’s most common for a subscript to take a single parameter, you can also define a subscript with multiple parameters if it’s appropriate for your type. The following example defines a Matrix
structure, which represents a two-dimensional matrix of Double
values. The Matrix
structure’s subscript takes two integer parameters:
添え字がただ1つのパラメータをとることが最も普通であるけれども、あなたはまた、それがあなたの型に適切ならば、複数のパラメータをもつ添え字を定義することができます。以下の例はMatrix
構造体を定義します、それはDouble
値からなる2次元行列を表します。Matrix
構造体の添え字は、2つの整数パラメータをとります:
- struct Matrix {
- let rows: Int, columns: Int
- var grid: [Double]
- init(rows: Int, columns: Int) {
- self.rows = rows
- self.columns = columns
- grid = Array(repeating: 0.0, count: rows * columns)
- }
- func indexIsValid(row: Int, column: Int) -> Bool {
- return row >= 0 && row < rows && column >= 0 && column < columns
- }
- subscript(row: Int, column: Int) -> Double {
- get {
- assert(indexIsValid(row: row, column: column), "Index out of range")
- return grid[(row * columns) + column]
- }
- set {
- assert(indexIsValid(row: row, column: column), "Index out of range")
- grid[(row * columns) + column] = newValue
- }
- }
- }
Matrix
provides an initializer that takes two parameters called rows
and columns
, and creates an array that’s large enough to store rows * columns
values of type Double
. Each position in the matrix is given an initial value of 0.0
. To achieve this, the array’s size, and an initial cell value of 0.0
, are passed to an array initializer that creates and initializes a new array of the correct size. This initializer is described in more detail in Creating an Array with a Default Value.
Matrix
はひとつのイニシャライザを提供します、それはrows
とcolumns
と呼ばれる2つのパラメータをとり、型Double
で個数rows * columns
の値を格納するのに十分大きい配列をつくります。行列の中の各位置は、0.0
の初期値を与えられます。これを達成するために、この配列の大きさ、そして0.0
の初期セル値は、正しいサイズの新しい配列をつくって初期化する配列イニシャライザに渡されます。このイニシャライザは、更に詳細に配列を1つの初期値で作成するで記述されます。
You can construct a new Matrix
instance by passing an appropriate row and column count to its initializer:
あなたは、そのイニシャライザに適切な行と列の数を渡すことによって新しいMatrix
インスタンスを造ることができます:
- var matrix = Matrix(rows: 2, columns: 2)
The example above creates a new Matrix
instance with two rows and two columns. The grid
array for this Matrix
instance is effectively a flattened version of the matrix, as read from top left to bottom right:
上の例は、2つの行と2つの列で新しいMatrix
インスタンスをつくります。このMatrix
インスタンスのためのgrid
配列は、実際にはこのMatrix 行列の、左上から右下へと読まれる、平らにされた改変板です:
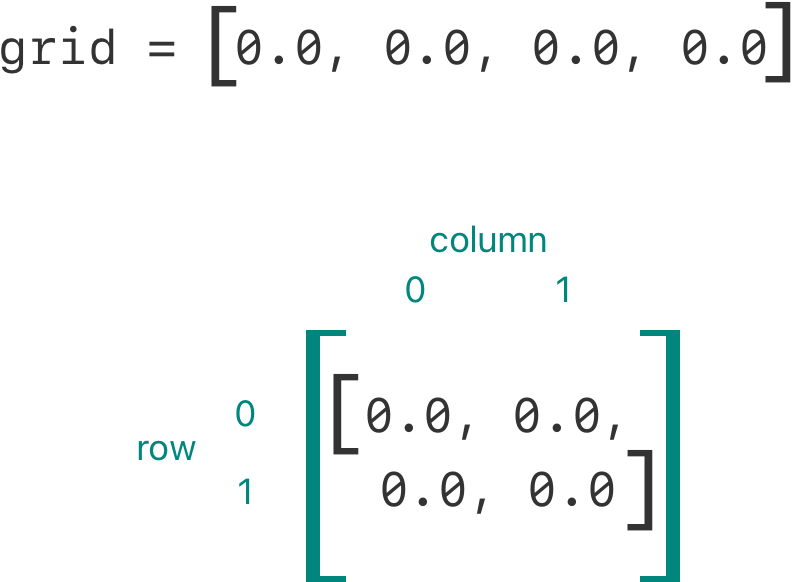
Values in the matrix can be set by passing row and column values into the subscript, separated by a comma: 行列の中の値は、コンマで区切った行と列の値を添え字に渡すことによって設定されることができます:
- matrix[0, 1] = 1.5
- matrix[1, 0] = 3.2
These two statements call the subscript’s setter to set a value of 1.5
in the top right position of the matrix (where row
is 0
and column
is 1
), and 3.2
in the bottom left position (where row
is 1
and column
is 0
):
これらの2つの文は、添え字のセッターを呼び出して、この行列の右上位置(row
が0
でcolumn
が1
のところ)に1.5
の値を、そして左下位置(row
が1
でcolumn
が0
のところ)に3.2
を設定します:
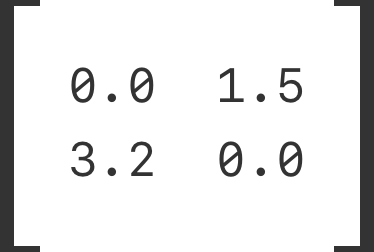
The Matrix
subscript’s getter and setter both contain an assertion to check that the subscript’s row
and column
values are valid. To assist with these assertions, Matrix
includes a convenience method called indexIsValid(row:column:)
, which checks whether the requested row
and column
are inside the bounds of the matrix:
Matrix
添え字のゲッターとセッターは両方とも、添え字のもつrow
とcolumn
値が有効なことを確認するためにひとつの表明を含みます。これらの表明を手伝うために、Matrix
はindexIsValid(row:column:)
と呼ばれるある便利なメソッドを含みます、それは要請されたrow
とcolumn
が行列の境界の内側にあるかどうか確認します:
- func indexIsValid(row: Int, column: Int) -> Bool {
- return row >= 0 && row < rows && column >= 0 && column < columns
- }
An assertion is triggered if you try to access a subscript that’s outside of the matrix bounds: あなたがマトリックス境界の外にある添え字にアクセスしようとするならば、表明が引き起こされます:
- let someValue = matrix[2, 2]
- // This triggers an assert, because [2, 2] is outside of the matrix bounds.(これはある表明の引き金となります、なぜなら [2, 2] はマトリックス境界の外であるからです。)
Type Subscripts¶ 型添え字¶
Instance subscripts, as described above, are subscripts that you call on an instance of a particular type. You can also define subscripts that are called on the type itself. This kind of subscript is called a type subscript. You indicate a type subscript by writing the static
keyword before the subscript
keyword. Classes can use the class
keyword instead, to allow subclasses to override the superclass’s implementation of that subscript. The example below shows how you define and call a type subscript:
インスタンス添え字は、上で記述されるように、あなたがある特定の型のインスタンス上で呼び出す添え字です。あなたはまた、型それ自身の上で呼び出される添え字を定義できます。この種の添え字は、型添え字と呼ばれます。あなたは型添え字を、static
キーワードをsubscript
キーワードの前に書くことによって指し示します。クラスは、class
キーワードを代わりに使用して、サブクラスにスーパークラスのもつその添え字の実装のオーバーライドを許可できます。下の例は、どのようにあなたが型添え字を定義して呼び出すかを示します:
- enum Planet: Int {
- case mercury = 1, venus, earth, mars, jupiter, saturn, uranus, neptune
- static subscript(n: Int) -> Planet {
- return Planet(rawValue: n)!
- }
- }
- let mars = Planet[4]
- print(mars)