PlaygroundPage Class
PlaygroundPageクラス
An object that represents the state of a playground page and enables you to interact with the Swift Playgrounds live view.
あるオブジェクト、それはプレイグラウンドページの状態を表して、あなたにSwift Playgroundsライブビューとの相互作用を可能にします。
Overview
概要
A PlaygroundPage
object provides methods and properties that represent the state of a playground page, enabling you to interact with Swift Playgrounds. Functionality includes displaying a live view, accessing the text in the playground, checking the assessment status, ending the execution of a playground, and accessing the live view.
PlaygroundPage
オブジェクトはメソッドとプロパティを提供します、それはプレイグラウンドページの状態を表して、あなたにSwift Playgroundsと相互作用させます。機能性は、ライブビューの表示、プレイグラウンドの中のテキストへのアクセス、評価状態の確認、プレイグラウンド実行の終了、そしてライブビューへのアクセスを含みます。
Class Properties
クラスプロパティ
current
The current playground page.
現在のプレイグラウンドページ。
Declaration
宣言
static let current: PlaygroundPage
Discussion
解説
Use current
to find the playground page instance.
current
を使ってプレイグラウンドページインスタンスを見つけてください。
Instance Properties
インスタンスプロパティ
assessmentStatus
The assessment status for the page, if any; otherwise nil
.
このページに対する評価状態、もしあれば;そうでなければnil
。
Declaration
宣言
var assessmentStatus: AssessmentStatus? { get set }
Discussion
解説
Set the assessment status to display success text or update the hints for the page.
結果成功テキストを表示またはページに対するヒントを更新するために、評価状態を設定します。
Assessment status for a page is initialized to nil
, indicating that the page has not been assessed.
あるページに対する評価状態はnil
に初期化されいて、そのページが評価され終わっていないことを指し示します。
For possible values, see AssessmentStatus Enumeration.
可能な値のために、AssessmentStatus列挙を見てください。
For example, to show the success text “You did it!” set the assessment status to .pass
, as shown below.
例えば、結果成功テキスト「You did it!」を表示するには評価状態を.pass
に設定してください、下に示すように。
PlaygroundPage.current.assessmentStatus = .pass(message: "You did it!")
liveView
The active live view, if any; otherwise nil
.
アクティプなライブビュー、もしあれば;そうでなければnil
。
Declaration
宣言
var liveView: PlaygroundLiveViewable? { get set }
Discussion
解説
Display a live view by setting liveView
to an object that conforms to the PlaygroundLiveViewable Protocol. The live view is displayed in the live view pane for the current playground page. There can be only one live view open at any time.
liveView
をPlaygroundLiveViewable Protocolプロトコルに準拠するオブジェクトに設定することによって、あるライブビューを表示してください。ライブビューは、現在のプレイグラウンドページのためのライブビューペーンにおいて表示されます。ライブビューはいつでもただ1つしか存在しません。
To open a live view, set PlaygroundPage.current.liveView
to an instance of a class that inherits from UIViewController
or UIView
. The live view can be opened from any source file and runs only while the playground is executing. When liveView is set to a non-nil
value, the system sets needsIndefiniteExecution
to true
. Dismiss an open live view by setting liveView
to nil
.
あるライブビューを開くには、PlaygroundPage.current.liveView
をUIViewController
またはUIView
から継承するクラスのインスタンスに設定してください。ライブビューは、どんなソースファイルからでも開かれることができ、プレイグラウンドが実行されている間のみ動作します。liveViewが非nil
値に設定される間、システムはneedsIndefiniteExecution
をtrue
に設定します。開いているライブビューを、liveView
をnil
に設定することで片付けてください。
Live views opened from LiveView.swift
start running when the playground is opened and do not require the playground to be executing. Setting liveView
to nil
does not dismiss an always-on live view. Message sending between an always-on live view and the playground page requires a proxy. For more information, see PlaygroundRemoteLiveViewProxy Class.
LiveView.swift
から開かれたライブビューは、プレイグラウンドが開かれる時に動作を始め、実行されるのにプレイグラウンドを必要としません。liveView
をnil
に設定することは、常時接続のライブビューを退去させません。常時接続のライブビューとプレイグラウンドページとの間でメッセージを送るには、プロキシを必要とします。詳細として、PlaygroundRemoteLiveViewProxyクラスを見てください。
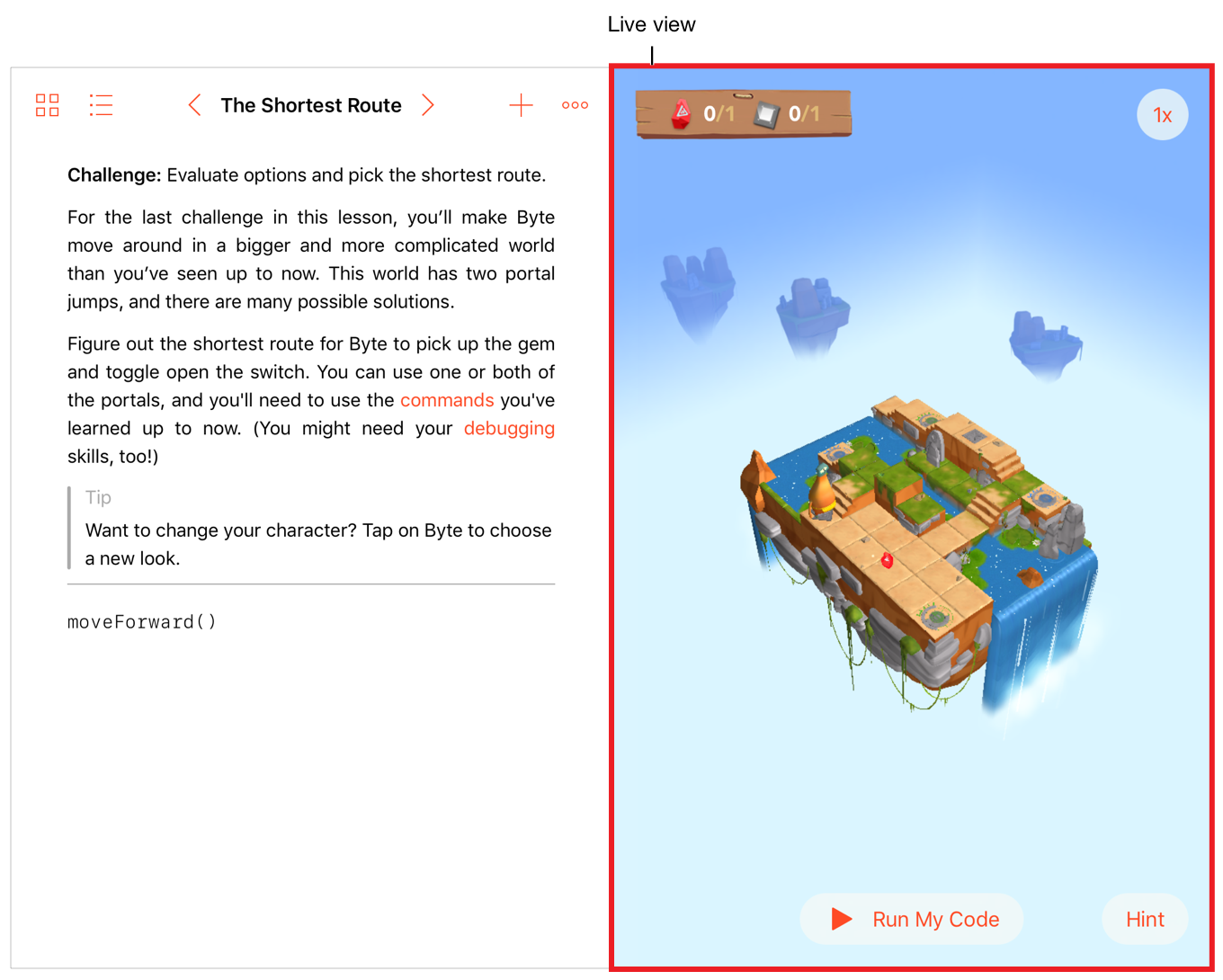
needsIndefiniteExecution
A Boolean value that indicates whether indefinite execution is enabled.
無期限の実行が可能にされるかどうかを指し示すブール値。
Declaration
宣言
var needsIndefiniteExecution: Bool { get set }
Discussion
解説
By default, all top-level code is executed, and then execution stops. When working with asynchronous code, enable indefinite execution to allow execution to continue after the end of the playground’s top-level code is reached. This, in turn, gives threads and callbacks time to execute.
初期状態で、すべてのトップレベルコードが実行されて、それから実行は停止します。非同期コードを扱う場合、無期限の実行を可能にすることで、プレイグラウンドのトップレベルコードの終わりに届いた後に、実行が継続できるようにしてください。これは、順番に、スレッドとコールバックに実行に十分な時間を与えます。
A user editing the playground or touching the Stop button stops execution automatically, even when indefinite execution is enabled.
プレイグラウンドを編集しているまたはStopボタンをタッチしているユーザは、自動的に実行を停止します、無期限実行が可能にされている時でさえもです。
Set needsIndefiniteExecution
to true
to continue execution after top-level code stops executing. Set it to false
before the end of the top-level code is reached to stop execution after top-level code stops. Setting it to false while the playground is executing continuously has no effect. To stop execution at any time, use finishExecution()
.
needsIndefiniteExecution
をtrue
に設定することで、トップレベルコードが実行を停止する後で実行を継続してください。それをトップレベルコードの終わりに届く前にfalse
に設定することで、トップレベルコードが停止する後に実行を停止してください。それをプレイグラウンドが実行している間にfalseに設定することは、効果を持ちません。いつでも実行を停止するには、 finishExecution()
を使ってください。
The default value is false
. It is set to true
when liveView is set to a non-nil value.
省略時の値は、false
です。それがtrue
に設定されるのは、liveViewが非nil値に設定される時です。
text
The current contents of the playground page, including any user-entered text.
プレイグラウンドページの現在の内容、あらゆるユーザ入力テキストを含みます。
Declaration
宣言
var text: String { get }
Discussion
解説
The following code checks if the text of the playground page contains one of the strings “llama” or “Llama”. If it does, a success dialog is presented.
以下のコードは、プレイグラウンドのテキストが文字列「llama」または「Llama」のうちの1つを含むかどうかを調べます。それがそうするならば、成功結果ダイアログが提示されます。
let pageText = PlaygroundPage.current.text
if pageText.contains("llama") || pageText.contains("Llama") {
PlaygroundPage.current.assessmentStatus = .pass(message: "That's the right animal")
}
Instance Methods
インスタンスメソッド
finishExecution()
Stops executing the current playground page.
現在のプレイグラウンドページの実行を停止します。
Declaration
宣言
func finishExecution() -> Never
PlaygroundKeyValueStore Class
PlaygroundKeyValueStoreクラス
Copyright © 2018 Apple Inc. All rights reserved. Terms of Use | Privacy Policy | Updated: 2018-04-30